数据结构(c语言版)编程创建一个含有n个节点的链表(不包含头节点),n由键盘输入,按照顺序存分别储数字1到n,使用递归的方法倒叙输出 效果如下: 输入:5 输出:5 4 3 2 1
时间: 2024-09-29 17:08:24 浏览: 41
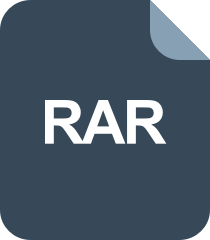
C语言实现键盘输入数据构建链表后冒泡排序

在C语言中,我们可以使用递归来创建一个链表并存储从1到n的数字,然后使用递归的方式反转链表来达到倒序输出的效果。这里是一个简单的示例:
首先,我们需要定义一个链表节点结构体:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
接着,创建链表的函数会采用递归形式:
```c
Node* createList(int n, int current = 1) {
if (current > n) {
return NULL; // 遇到边界条件,返回空指针
}
Node* newNode = (Node*)malloc(sizeof(Node)); // 分配内存
newNode->data = current;
newNode->next = createList(n, current + 1); // 递归调用,将当前值加一
return newNode;
}
```
最后,我们编写一个辅助函数用于递归地反转链表:
```c
void reverseList(Node** head) {
if (*head == NULL || (*head)->next == NULL) { // 基线条件:单个元素或空链表
return;
}
Node* temp = (*head)->next; // 保存下一个节点
(*head)->next = NULL; // 简化反转后的链接
reverseList(&temp); // 递归处理剩余部分
(*head)->next = temp; // 将反转后的节点连接回原链表
}
// 主程序入口
int main() {
int n;
printf("请输入链表长度:");
scanf("%d", &n);
Node* list = createList(n);
// 反转链表
reverseList(&list);
Node* currentNode = list;
while (currentNode != NULL) {
printf("%d ", currentNode->data);
currentNode = currentNode->next;
}
free(list); // 释放内存
return 0;
}
```
当你运行这个程序并输入5时,它会按照所描述的方式输出结果:`5 4 3 2 1`。
阅读全文
相关推荐
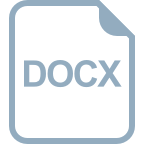
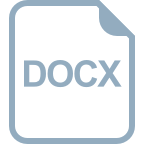


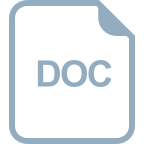
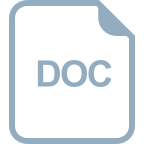
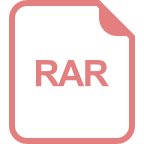
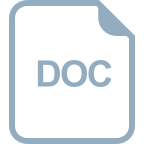
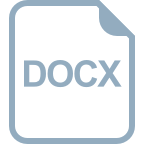
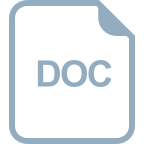
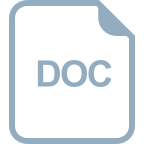
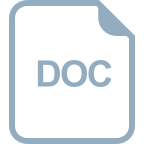
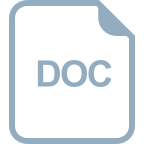
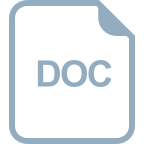
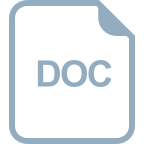
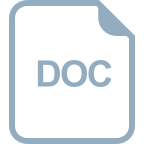
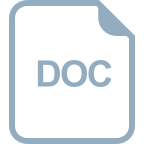

