TPM2.0 AES加密 C#实现
时间: 2024-10-12 16:11:08 浏览: 24
TPM(Trusted Platform Module),即可信平台模块,是一个安全芯片,用于增强系统的安全性。AES(Advanced Encryption Standard)是一种高级加密标准,常用于数据加密。在C#中实现TPM2.0的AES加密通常需要借助第三方库,如TPM.NET等,因为C#本身并未直接支持TPM。
以下是一个简单的示例,展示如何使用第三方库TPM.NET进行AES加密操作:
```csharp
using System;
using TpmNet;
public class TpmAesExample
{
public static void Main(string[] args)
{
// 初始化TPM设备
var tpm = TpmManager.Instance.Initialize();
try
{
// 加载密钥对
using (var keyBlob = new TpmBuffer(new byte[] { /* 插入你的密钥数据 */ }))
{
var loadedKey = LoadKey(tpm, keyBlob);
// 创建AES上下文
var aesAlg = new AesContext(loadedKey);
// 设置明文和密文块大小
var blockSize = aesAlg.BlockSize;
var input = "This is a test string".ToBytes();
var output = new byte[input.Length];
// 加密
if (aesAlg.Encrypt(input, output))
{
Console.WriteLine("Original text:");
Console.WriteLine(Encoding.UTF8.GetString(input));
Console.WriteLine("Encrypted text:");
Console.WriteLine(Encoding.UTF8.GetString(output));
}
else
{
Console.WriteLine("Encryption failed.");
}
}
}
finally
{
// 关闭TPM资源
tpm.Shutdown();
}
}
private static TpmHandle LoadKey(TpmManager tpm, TpmBuffer keyBlob)
{
// 实际的TPM加载函数,这取决于TPM.NET的具体API
// 这里假设存在一个LoadKey方法
return tpm.LoadKey(keyBlob, out _);
}
}
```
注意,这只是一个基础示例,实际应用中你可能需要处理更多的错误情况,并确保TPM.NET库的正确配置和安装。同时,TPM操作通常是硬件相关的,可能会受限于特定硬件的支持。
阅读全文
相关推荐
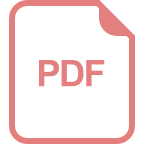
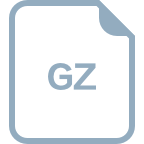

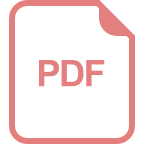
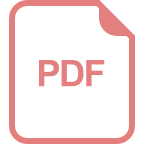
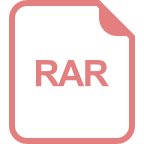
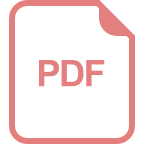
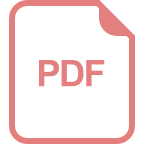
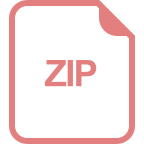
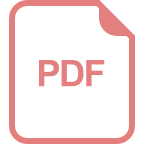
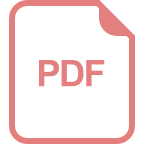
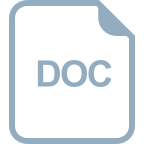
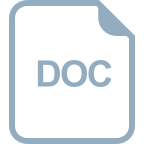
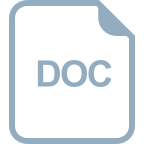



