这个脚本运行后如果服务端未打开连接,即使程序停止了,ConnectAsync方法仍旧一直检测连接,该如何优化这个问题
时间: 2024-11-23 14:46:15 浏览: 22
为了优化 `ConnectAsync` 方法在服务端未打开连接时持续检测的问题,可以采取以下几种措施:
1. **增加超时机制**:设置一个合理的重连尝试次数或时间限制,避免无限次重连。
2. **引入取消令牌**:使用 `CancellationToken` 来控制重连逻辑,当需要停止重连时可以通过取消令牌来中断任务。
3. **改进日志记录和状态管理**:更好地记录重连的状态,并提供更详细的日志信息以便调试。
以下是优化后的代码示例:
```csharp
public class TCPClient : MonoBehaviour
{
private TcpClient tcpClient;
private NetworkStream networkStream;
private string serverIp;
private int serverPort;
private bool isReconnecting;
private CancellationTokenSource cancellationTokenSource;
private int maxRetryAttempts = 5; // 最大重试次数
private int retryAttempt = 0; // 当前重试次数
// 其他成员变量和构造函数保持不变
public void Init()
{
serverIp = DataManager.TCPIP;
serverPort = int.Parse(DataManager.TCPPort);
OnConnected += ConnectTcp;
InvokeRepeating("CheckConnectAsync", 5, 10);
cancellationTokenSource = new CancellationTokenSource();
}
public async Task ConnectAsync()
{
try
{
tcpClient = new TcpClient();
isReconnecting = false;
await tcpClient.ConnectAsync(serverIp, serverPort);
OnConnected?.Invoke();
networkStream = tcpClient.GetStream();
StartReceiving();
// 保持连接
while (tcpClient.Connected)
{
await Task.Delay(1000);
}
}
catch (Exception e)
{
OnDisconnected?.Invoke();
Debug.LogError($"连接失败: {e.Message}");
if (!isReconnecting && retryAttempt < maxRetryAttempts)
{
isReconnecting = true;
retryAttempt++;
await Task.Delay(5000); // 等待5秒后重连
await ConnectAsync();
}
else
{
Debug.LogWarning("达到最大重试次数,停止重连。");
cancellationTokenSource.Cancel();
}
}
}
// 其他方法保持不变
public void Disconnect()
{
if (tcpClient != null)
{
tcpClient.Close();
OnDisconnected?.Invoke();
}
cancellationTokenSource?.Cancel();
retryAttempt = 0; // 重置重试次数
}
async Task CheckConnectAsync()
{
if (!tcpClient.Connected && !isReconnecting && retryAttempt < maxRetryAttempts)
{
isReconnecting = true;
await ConnectAsync();
}
}
private void OnDestroy()
{
Disconnect();
CancelInvoke("CheckConnectAsync");
}
}
```
### 关键改动点:
1. **增加最大重试次数**:通过 `maxRetryAttempts` 和 `retryAttempt` 变量来控制重连的最大次数。
2. **引入取消令牌**:在 `Init` 方法中创建 `cancellationTokenSource`,并在 `Disconnect` 方法中取消它,以确保在不需要重连时能够及时终止重连任务。
3. **改进日志记录**:在达到最大重试次数时输出警告日志,提示用户已停止重连。
这样可以有效地避免在服务端未打开连接时无限次重连的问题。
阅读全文
相关推荐



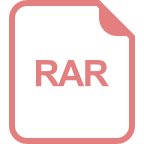




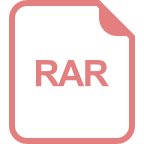









