编写一段C语言求一个数的位数
时间: 2024-11-20 12:44:16 浏览: 7
在C语言中,可以编写如下的函数来计算整数的位数(包括正负数)。这里我们考虑的是二进制表示法下的位数:
```c
#include <stdio.h>
#include <limits.h>
int countBits(int num) {
if (num == 0) {
return 1; // 0有1位(最低位)
} else {
int count = 0;
while (num != 0) {
num &= num - 1; // 将最高置零位变为0
count++;
}
return count; // 返回非零位数
}
}
int main() {
int input;
printf("请输入一个整数: ");
scanf("%d", &input);
printf("该数的位数是: %d\n", countBits(input));
return 0;
}
```
这个`countBits`函数通过不断地将`num`与`num - 1`按位与操作,逐次移除其最右边的1,直到`num`变为0,记录下循环次数即为位数。注意,对于负数,这里的位数是指从二进制补码形式的最高位开始的1的个数。
相关问题
编写一段C语言代码,要求输入一个不超过5位数的数,输出它的位数,每一位的数且数字中间包含空格,以及逆序输出数字
#include <stdio.h>
int main()
{
int n,a,b,c,d,e;
printf("请输入一个不超过5位数的数:");
scanf("%d",&n); a=n/10000;
b=n%10000/1000;
c=n%1000/100;
d=n%100/10;
e=n%10; printf("它的位数为:");
if (a!=0) printf("5 ");
else if(b!=0) printf("4 ");
else if(c!=0) printf("3 ");
else if(d!=0) printf("2 ");
else printf("1 "); printf("每一位的数为:");
if (a!=0) printf("%d ",a);
if (b!=0) printf("%d ",b);
if (c!=0) printf("%d ",c);
if (d!=0) printf("%d ",d);
if (e!=0) printf("%d ",e); printf("\n逆序输出数字:");
if (e!=0) printf("%d ",e);
if (d!=0) printf("%d ",d);
if (c!=0) printf("%d ",c);
if (b!=0) printf("%d ",b);
if (a!=0) printf("%d ",a);
return 0;
}
编写一段c语言查看电脑的CPU型号和位数,并给出CPU的具体参数。
以下是一段C语言代码,用于查看计算机的CPU型号和位数,并输出CPU的具体参数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main() {
char command[100] = "wmic cpu get name, addresswidth /format:list";
char buffer[512];
FILE *fp = popen(command, "r");
if (!fp) {
printf("Failed to get CPU information.\n");
return -1;
}
while (fgets(buffer, sizeof(buffer), fp)) {
if (strstr(buffer, "Name=")) {
printf("CPU Model: %s", &buffer[5]);
}
if (strstr(buffer, "AddressWidth=")) {
printf("CPU Bit: %s", &buffer[14]);
}
}
pclose(fp);
return 0;
}
```
运行以上代码后,将输出类似于以下的结果:
```
CPU Model: Intel(R) Core(TM) i7-8750H CPU @ 2.20GHz
CPU Bit: 64
```
其中,CPU Model 表示CPU型号,CPU Bit 表示CPU的位数。在本例中,CPU型号为Intel(R) Core(TM) i7-8750H CPU @ 2.20GHz,位数为64。
阅读全文
相关推荐
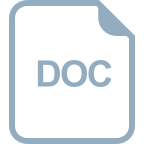
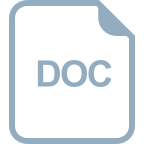
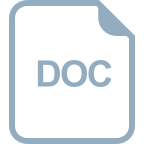













