用c语言实现 ListInsert(Node *head, unsigned int index, DataType e):在链表给定的位置(0---N-1)插入元素
时间: 2024-09-18 15:01:15 浏览: 37
在C语言中,要实现链表`ListInsert`函数,通常会涉及到以下几个步骤:
1. 检查头节点是否为空(即链表是否为空):
```c
if (head == NULL) {
head = (Node*)malloc(sizeof(Node)); // 如果为空,创建新节点作为头节点
head->data = e; // 设置数据
head->next = NULL;
}
```
2. 如果链表非空,处理插入操作:
- 定义一个新的节点,存储待插入的数据 `DataType e`
- 初始化新节点的 `next` 指针为 `NULL`
- 计算需要插入元素的新位置 `temp = head`
- 遍历链表到指定索引 `index`:
```c
for (unsigned int i = 0; i < index && temp != NULL; i++) {
temp = temp->next;
}
```
3. 插入新节点:
- 将新节点设置为当前节点的下一个节点:
```c
if (temp == NULL) { // 如果已经到达链表末尾,将新节点设为尾节点
temp->next = (Node*)malloc(sizeof(Node));
temp->next->data = e;
temp->next->next = NULL;
} else {
temp->next = (Node*)malloc(sizeof(Node));
temp->next->data = e;
temp->next->next = temp->next;
}
```
完整的`ListInsert`函数可能看起来像这样:
```c
void ListInsert(Node** head, unsigned int index, DataType e) {
Node* temp = *head;
if (*head == NULL) {
*head = (Node*)malloc(sizeof(Node));
(*head)->data = e;
(*head)->next = NULL;
} else {
while (temp != NULL && index > 0) {
temp = temp->next;
index--;
}
if (temp == NULL) { // 插入在链表末尾
temp->next = (Node*)malloc(sizeof(Node));
temp->next->data = e;
temp->next->next = NULL;
} else {
temp->next = (Node*)malloc(sizeof(Node));
temp->next->data = e;
temp->next->next = temp->next;
}
}
}
```
阅读全文
相关推荐
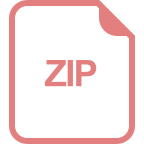
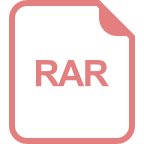
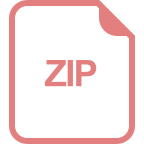






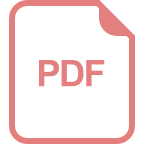
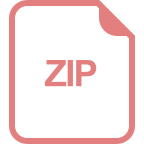
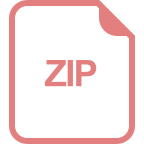
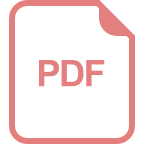
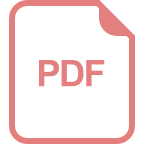
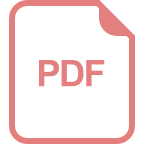
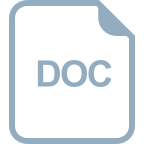
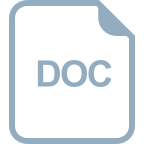

