python 读取二进制文件 np.array
时间: 2023-11-20 19:59:44 浏览: 62
以下是使用numpy读取二进制文件的例子:
```python
import numpy as np
# 读取二进制文件
myarray = np.fromfile("./query_feature_A/00056451.dat", dtype=float)
# 打印数组长度和每个元素
print("len(myarray)::", myarray.size)
for item in myarray:
print(item)
# 另一种读取二进制文件的方法
import struct
import os
fpath='./data.bin'
f = open(fpath,'rb')
nums=int(os.path.getsize(fpath)/4) # 4 是浮点数字节
data = struct.unpack('f'*nums,f.read(4*nums)) #注意一次只能读取一次,直接再读取会报错
f.close()
data = np.array(data).reshape(-1)
```
相关问题
python scipy二进制文件读取
要在 Python 中使用 Scipy 读取二进制文件,您可以使用 `numpy` 和 `scipy.io` 模块的函数。以下是一个读取二进制文件的示例代码:
```python
import numpy as np
from scipy.io import loadmat
# 读取二进制文件
data = loadmat('binary_data.mat')
# 获取数据数组
binary_array = data['binary_array']
# 打印数组内容
print(binary_array)
```
在这个示例中,我们首先导入了 `numpy` 和 `scipy.io` 模块。然后使用 `loadmat` 函数加载二进制文件,并将其保存在 `data` 变量中。接下来,我们从 `data` 中获取名为 `binary_array` 的数组,并将其保存在 `binary_array` 变量中。最后,打印出 `binary_array` 数组的内容。
这是一个基本的示例,您可以根据您的实际需求对代码进行修改。Scipy 的 `loadmat` 函数还提供了其他参数和选项,可以根据您的需求进行设置。您可以参考 Scipy 的官方文档以获取更多关于 `loadmat` 函数的详细信息。
python读取二进制图片
要读取二进制图片,你可以使用Python的内置模块PIL(Python Imaging Library)或者OpenCV库。下面是使用PIL库读取二进制图片的示例代码:
```python
from PIL import Image
import io
# 打开二进制图片文件
with open('image.jpg', 'rb') as f:
# 创建一个BytesIO对象,并将文件内容写入到该对象中
bytes_io = io.BytesIO(f.read())
# 使用PIL库打开BytesIO对象中的图片
image = Image.open(bytes_io)
# 可以对图片进行进一步的操作,比如显示、保存等
image.show()
```
在上面的示例中,我们首先使用`open`函数打开二进制图片文件,并以二进制模式`'rb'`读取文件内容。然后,我们创建一个`BytesIO`对象,并将文件内容写入到该对象中。接下来,使用`Image.open`函数打开`BytesIO`对象中的图片。最后,你可以对图片进行进一步的操作,比如显示(`image.show()`)或保存(`image.save('output.jpg')`)。
注意,你需要将`image.jpg`替换为你实际的图片文件路径。
如果你想使用OpenCV库读取二进制图片,你可以使用`imdecode`函数来实现。下面是使用OpenCV库读取二进制图片的示例代码:
```python
import cv2
import numpy as np
# 读取二进制图片文件
with open('image.jpg', 'rb') as f:
# 读取文件内容
image_data = f.read()
# 将二进制数据转换为numpy数组
np_array = np.frombuffer(image_data, np.uint8)
# 使用OpenCV库将numpy数组解码为图片
image = cv2.imdecode(np_array, cv2.IMREAD_COLOR)
# 可以对图片进行进一步的操作,比如显示、保存等
cv2.imshow('Image', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的示例中,我们首先使用`open`函数打开二进制图片文件,并读取文件内容。然后,使用`frombuffer`函数将二进制数据转换为numpy数组。接下来,使用`imdecode`函数将numpy数组解码为图片。最后,你可以对图片进行进一步的操作,比如显示(`cv2.imshow`)或保存(`cv2.imwrite`)。
同样地,你需要将`image.jpg`替换为你实际的图片文件路径。
相关推荐
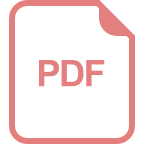
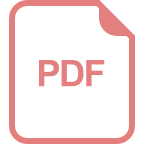












