c语言gethostbyname,实现超时返回的gethostbyname函数
时间: 2024-12-26 09:28:08 浏览: 14
`gethostbyname`是C标准库中的一个函数,用于将域名解析为IP地址。然而,这个函数本身并不支持超时功能,因为它是一个阻塞操作,一旦开始查询就会一直等待直到网络响应为止。如果你需要实现在一定时间内未获得结果就返回的功能,你需要自定义一个封装。
下面是一个简单的示例,展示如何使用`gethostbyname`配合定时器来实现超时版本:
```c
#include <stdio.h>
#include <stdlib.h>
#include <arpa/inet.h>
#include <netdb.h>
#include <sys/socket.h>
#include <unistd.h>
#include <signal.h> // 用于处理信号
// 自定义结构体,存储主机信息和超时时间
typedef struct {
struct hostent* host_info;
time_t timeout;
} HostByNameResult;
void timeout_handler(int signum) {
// 超时处理,这里简单地释放已分配的内存并设置错误标志
if (HostByNameResult.host_info != NULL) {
freeaddrinfo(HostByNameResult.host_info);
HostByNameResult.host_info = NULL;
}
printf("Timeout occurred.\n");
}
int get_host_by_name_with_timeout(char* hostname, int timeout_seconds) {
HostByNameResult result;
result.timeout = time(NULL) + timeout_seconds; // 设置超时时间
signal(SIGALRM, timeout_handler); // 注册超时信号处理程序
alarm(timeout_seconds);
result.host_info = gethostbyname(hostname);
alarm(0); // 关闭超时计时器
return result.host_info != NULL ? 0 : -1; // 如果成功返回NULL,失败则返回-1
}
int main() {
char* domain = "example.com";
int timeout = 5; // 5秒超时
int ret = get_host_by_name_with_timeout(domain, timeout);
if (ret == 0) {
printf("Host found: %s\n", inet_ntoa(*(struct in_addr*)result.host_info->h_addr_list[0]));
} else {
printf("Failed to resolve host within %d seconds or timed out.\n", timeout);
}
return 0;
}
```
这个示例中,我们通过注册一个信号处理器来触发超时。当时间到达指定的超时时限,会发送一个SIGALRM信号,然后在`timeout_handler`中处理,如果这时仍未完成解析,那么会释放资源并返回错误。
注意:这只是一个简化版的实现,并不是真正的高精度超时,因为系统信号处理可能会有额外的延迟。在实际生产环境中,可能需要使用更专业的网络库或第三方工具来实现更精确的超时控制。
阅读全文
相关推荐







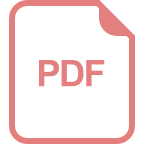









