C++自行编写strcmp
时间: 2023-12-02 18:41:15 浏览: 65
根据提供的引用内容,我们可以了解到评测机是用纯C++编写的,因此可以自行编写strcmp函数。下面是一个简单的strcmp函数的实现:
```c++
int my_strcmp(const char* str1, const char* str2) {
while (*str1 && *str2 && (*str1 == *str2)) {
str1++;
str2++;
}
return *str1 - *str2;
}
```
该函数的实现思路是:逐个比较两个字符串中的字符,如果相同则继续比较下一个字符,直到出现不同的字符或者到达字符串结尾。如果两个字符串完全相同,则返回0;如果str1大于str2,则返回正数;如果str1小于str2,则返回负数。
相关问题
c++ strcmp
C++中的strcmp函数用于比较两个字符串的大小。它接受两个C风格的字符串作为参数,并返回一个整数值来表示比较结果。
strcmp函数的原型如下:
```cpp
int strcmp(const char* str1, const char* str2);
```
其中,str1和str2分别是要比较的两个字符串。
strcmp函数返回的整数值有以下几种可能的情况:
- 若str1小于str2,则返回一个负数;
- 若str1等于str2,则返回0;
- 若str1大于str2,则返回一个正数。
具体的比较规则是根据ASCII码值来确定的。对于每一个字符,strcmp函数会逐个比较它们的ASCII码值,直到找到不同的字符或者遇到字符串结束符'\0'为止。
下面是一个使用strcmp函数比较两个字符串的示例:
```cpp
#include <iostream>
#include <cstring>
int main() {
const char* str1 = "Hello";
const char* str2 = "World";
int result = std::strcmp(str1, str2);
if (result < 0) {
std::cout << "str1 < str2" << std::endl;
} else if (result == 0) {
std::cout << "str1 == str2" << std::endl;
} else {
std::cout << "str1 > str2" << std::endl;
}
return 0;
}
```
运行上述代码,输出结果为"str1 < str2",表示"Hello"小于"World"。
用c语言编写strcmp算法
下面是用C语言编写的strcmp算法:
```c
int strcmp(const char *s1, const char *s2) {
while (*s1 && (*s1 == *s2)) {
s1++;
s2++;
}
return *(const unsigned char*)s1 - *(const unsigned char*)s2;
}
```
该算法的实现方式是:首先比较两个字符串的第一个字符,如果相等,则继续比较下一个字符,直到遇到不相等的字符或者其中一个字符串结束。如果两个字符串相等,则返回0,如果不相等,则返回两个字符串第一个不相等字符的差值。
注意,由于strcmp函数返回值是int类型,因此需要将字符的ASCII码值转换为无符号整型。
相关推荐
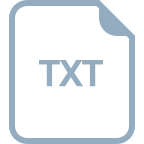












