用 C 语言不使用 strtok 函数实现将字符串192.168.1.101用分隔符.分割的代码:
时间: 2024-09-29 15:12:52 浏览: 44
在C语言中,如果你不希望使用`strtok()`函数,你可以选择手动遍历整个字符串,逐字符查找分隔符并记录子串。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 自定义分割字符串函数
void split_string(const char *str, const char delimiter, char **result) {
int index = 0;
*result = (char*)malloc(sizeof(char)*5); // 初始化结果数组的第一个元素
char temp[100]; // 临时存储子串
while (*str != '\0') {
if (*str == delimiter) { // 如果找到分隔符
(*result)[index] = '\0'; // 结束当前子串
index++; // 更新结果数组索引
result[index] = temp; // 将临时子串复制到结果数组
temp[0] = '\0'; // 清空临时子串
} else {
temp[index++] = *str; // 非分隔符时,直接添加到临时子串
}
str++;
}
// 最后一个子串不需要加'\0'
if(temp[index - 1] != '\0') {
strncpy(result[index], temp, strlen(temp));
}
}
int main() {
char str[] = "192.168.1.101";
char *ip_parts[4];
split_string(str, '.', ip_parts);
for(int i = 0; i < 4; i++) {
printf("IP部分 %d: %s\n", i+1, ip_parts[i]);
}
//记得释放内存
for(int i = 0; i < 4; i++) {
free(ip_parts[i]);
}
free(ip_parts);
return 0;
}
```
在这个例子中,我们创建了一个`split_string`函数,它接受一个字符串、分隔符和结果数组指针。函数遍历输入字符串,每当遇到分隔符时,就将之前的部分存入结果数组,并继续处理下一个部分。
阅读全文
相关推荐
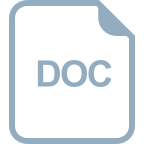
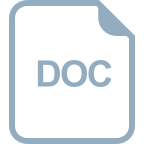
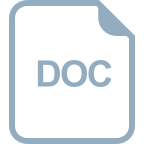
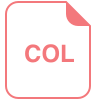

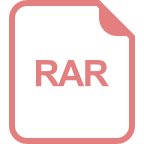
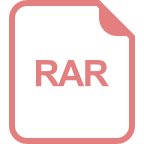






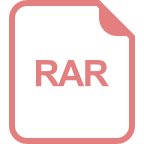




