二叉树中序非递归遍历代码
时间: 2024-11-06 19:19:55 浏览: 24
在二叉树的非递归中序遍历中,通常会利用栈数据结构来实现。下面是使用Python的代码示例:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
if root is None:
return []
stack, output = [], []
current = root
while True:
# 如果当前节点不为空,压入栈并移动到左子树
while current is not None:
stack.append(current)
current = current.left
# 如果栈不为空,弹出顶部节点,并添加其值到结果中,然后移到右子树
if stack:
current = stack.pop()
output.append(current.val)
current = current.right
# 当前节点和所有子节点都访问完,说明遍历结束
else:
break
return output
# 示例:
# 1
# / \
# 2 3
# / \
# 4 5
root = TreeNode(1, TreeNode(2, TreeNode(4), TreeNode(5)), TreeNode(3))
print(inorder_traversal(root)) # 输出:[4, 2, 5, 1, 3]
```
阅读全文
相关推荐
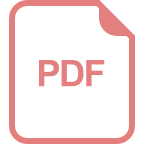
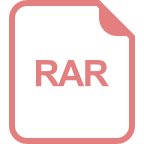
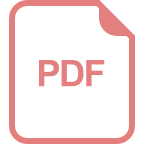















