vue2使用three.js
时间: 2023-10-30 14:07:54 浏览: 50
在Vue2中使用Three.js相对简单。首先,你需要在Vue项目中安装Three.js库。你可以使用npm或yarn来安装它。然后,你可以在组件中引入Three.js并创建场景(Scene)和渲染器(WebGLRenderer)。在Vue的生命周期钩子中,你可以使用render方法将渲染器的输出插入到DOM中。接下来,你可以使用Three.js提供的API来创建并操作三维对象。
相关问题
vue如何使用three.js
Vue 是一个流行的 JavaScript 框架,可以方便地与 Three.js 集成。您可以通过三个步骤来使用 Three.js:
1. 安装 Three.js:您可以使用 npm 安装 Three.js,或者在头部引入以下链接:
```
<script src="https://cdnjs.cloudflare.com/ajax/libs/three.js/r128/three.min.js"></script>
```
2. 创建场景和相机:使用 Three.js,您需要先创建场景和相机。
```
var scene = new THREE.Scene();
var camera = new THREE.PerspectiveCamera( fov, aspect, near, far );
```
3. 添加对象和渲染器:最后,您可以将对象添加到场景中,创建渲染器并将其添加到页面中。
```
var renderer = new THREE.WebGLRenderer();
document.body.appendChild( renderer.domElement );
renderer.render( scene, camera );
```
希望这可以帮助您开始使用 Three.js!
vue2如何使用three.js
您好,关于vue2如何使用three.js,您可以通过以下步骤添加three.js到vue2项目中:
1. 在项目中安装three.js库:npm install three
2. 在需要使用three.js的组件中,使用import语句引入three.js库: import * as THREE from 'three';
3. 创建一个THREE.Scene和THREE.Camera,并将Camera加入到Scene中。
4. 创建需要展示的物体,例如一个THREE.CubeGeometry对象、THREE.Mesh对象。
5. 在需要渲染的组件中,使用Three.js提供的渲染器THREE.WebGLRenderer,将Scene和Camera添加到渲染器中,再通过animate函数渲染动画。
详细的代码实现可以参考官方文档或者示例代码。希望可以帮助您。
相关推荐
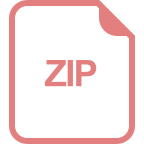












