实现线性表和单链表的插入、删除、查找等功能
时间: 2024-10-28 07:10:24 浏览: 22
在C语言中,线性表(数组)和单链表都是常见的数据结构,它们各自有不同的特点和操作方法。
**一、数组实现线性表**
1. 插入操作:
- **在数组末尾插入**: 如果有足够的空间,只需将最后一个元素后移一位,新元素放在末尾。
```c
void insert_array(int arr[], int n, int value) {
if (n < MAX_SIZE) { // 假设MAX_SIZE为数组的最大容量
arr[n] = value;
n++;
} else {
printf("Array is full.");
}
}
```
2. 删除操作:
- **删除指定位置的元素**: 需要移动其他元素填充空缺。
```c
void delete_array(int arr[], int n, int index) {
if (index >= 0 && index < n) {
for (int i = index; i < n - 1; i++) {
arr[i] = arr[i + 1];
}
n--;
} else {
printf("Invalid index.");
}
}
```
3. 查找操作:
- **查找特定值的位置**: 遍历整个数组进行比较。
```c
int search_array(int arr[], int n, int value) {
for (int i = 0; i < n; i++) {
if (arr[i] == value)
return i;
}
return -1; // 表示未找到
}
```
**二、单链表实现线性表**
1. 插入操作:
- **在头部插入**: 创建一个新的节点,然后链接到头节点前。
```c
struct Node* insert_at_start(struct Node* head, int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = head;
head = newNode;
return head;
}
```
- **在尾部插入**: 遍历链表找到最后一个节点,然后添加新节点。
```c
void insert_at_end(struct Node** head, int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = value;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
struct Node* temp = *head;
while (temp->next != NULL)
temp = temp->next;
temp->next = newNode;
}
```
2. 删除操作:
- **删除特定节点**: 找到并替换要删除节点的前驱节点,使其指向下一个节点。
```c
void delete_node(struct Node** head, int key) {
struct Node* temp = *head, *prev;
if (temp != NULL && temp->data == key) {
*head = temp->next;
free(temp);
return;
}
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL)
return;
prev->next = temp->next;
free(temp);
}
```
3. 查找操作:
- **查找特定值的节点**: 遍历链表逐个比较节点数据。
```c
struct Node* search_node(struct Node* head, int value) {
struct Node* current = head;
while (current != NULL) {
if (current->data == value)
return current;
current = current->next;
}
return NULL;
}
```
阅读全文
相关推荐
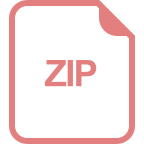
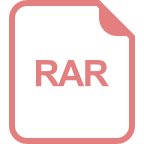
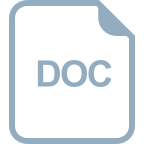
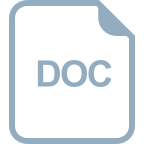
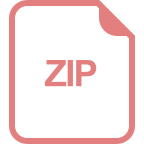
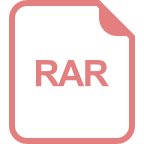
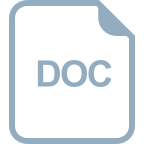
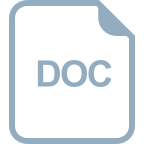
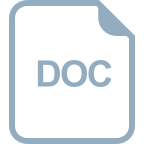
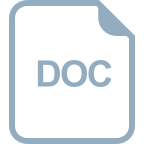
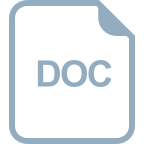
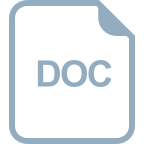
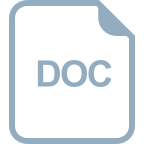
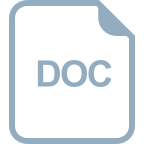
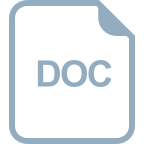
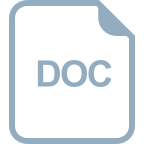
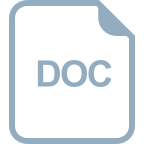