如何用Python编写一个函数来计算并返回字符串中每个字符及其连续出现的次数?
时间: 2024-09-10 10:29:44 浏览: 75
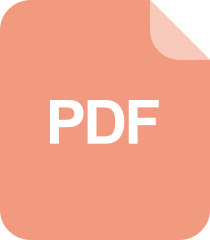
Python实现计算字符串中出现次数最多的字符示例
要用Python编写一个函数来计算并返回字符串中每个字符及其连续出现的次数,可以采用以下步骤:
1. 初始化一个空字典来存储字符和它们的连续出现次数。
2. 遍历字符串中的每个字符。
3. 在遍历过程中,检查当前字符是否与前一个字符相同。
4. 如果相同,则在字典中将该字符的计数加一。
5. 如果不同,则将该字符及其当前计数添加到字典中,并重置计数为1。
6. 遍历完成后,确保将最后一个字符及其计数添加到字典中(因为最后一个字符不需要与下一个字符比较)。
下面是实现上述步骤的Python函数示例:
```python
def count_consecutive_characters(input_string):
if not input_string:
return {}
char_count = {}
current_char = input_string[0]
count = 1
for char in input_string[1:]:
if char == current_char:
count += 1
else:
char_count[current_char] = count
current_char = char
count = 1
char_count[current_char] = count
return char_count
# 示例使用
input_str = "aaabbcccdefffg"
result = count_consecutive_characters(input_str)
print(result) # 输出应为 {'a': 3, 'b': 2, 'c': 3, 'd': 1, 'e': 1, 'f': 3, 'g': 1}
```
阅读全文
相关推荐
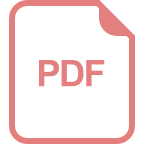
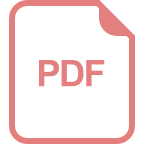















