一元线性回归梯度下降算法Python实现
时间: 2024-12-25 16:32:54 浏览: 7
一元线性回归梯度下降算法是一种常用的机器学习优化技术,用于求解最小化预测函数与实际值之间误差平方和的问题。在Python中,我们可以利用numpy库来进行简单实现。以下是基本步骤:
```python
import numpy as np
def gradient_descent(x, y, theta, learning_rate, num_iterations):
# 初始化权重和偏置
m = len(y) # 样本数
x_b = np.c_[np.ones((m, 1)), x] # 添加x的常数项
cost_history = []
for iteration in range(num_iterations):
# 前向传播计算预测值和误差
z = np.dot(x_b, theta)
error = y - z
# 计算梯度
gradient = (1/m) * np.dot(x_b.T, error)
# 更新权重
theta = theta + learning_rate * gradient
# 记录成本
cost = ((1/(2*m)) * np.sum(error**2))
cost_history.append(cost)
return theta, cost_history
# 示例数据
x_data = np.array([[1], [2], [3]])
y_data = np.array([2, 4, 5])
theta_initial = np.zeros(2) # 初始权重向量
learning_rate = 0.01
num_iterations = 1000
theta, cost_history = gradient_descent(x_data, y_data, theta_initial, learning_rate, num_iterations)
```
在这个例子中,`x_data`是特征矩阵,`y_data`是目标变量,`theta`是要更新的权重,`learning_rate`是步长,`num_iterations`是迭代次数。通过反复更新权重并计算代价函数,我们逐步接近最优解。
阅读全文
相关推荐
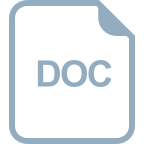
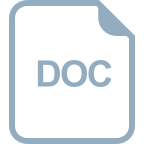
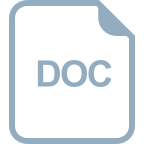
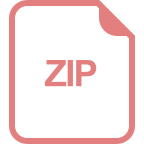
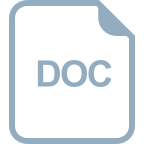
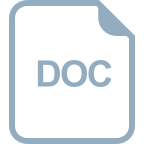

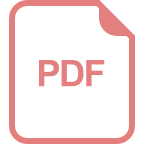
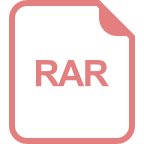
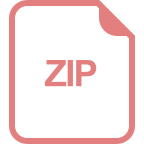
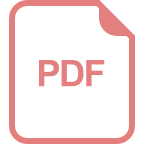
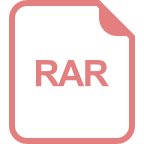
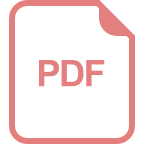
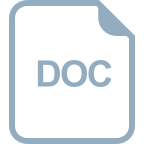


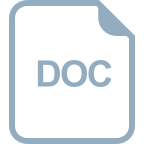
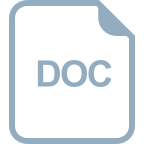
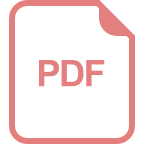
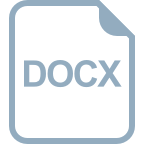