.编写一个利用线程互斥锁解决线程互斥的程序。要求父进程创建两个子线程,父进程和两个子线程模拟3个售票窗口进行售卖15张从青城山到犀浦的火车票,直到售完为止。假设1个窗口售卖1张火车票的时间为1秒。
时间: 2024-12-19 16:25:01 浏览: 8
为了实现这个场景,我们可以使用C++的`std::mutex`来保护共享资源,确保只有单个线程能够访问售票过程。这是一个简单的示例程序:
```cpp
#include <iostream>
#include <vector>
#include <thread>
#include <mutex>
std::mutex ticket_mutex; // 锁定对象,用于保护售票过程
const int total_tickets = 15;
const std::string destination = "犀浦";
int tickets_left = total_tickets;
void sell_ticket(int window_id) {
while (tickets_left > 0) {
std::lock_guard<std::mutex> lock(ticket_mutex); // 获取锁
if (tickets_left > 0) {
std::cout << "Window " << window_id << ": Selling ticket to " << destination << "\n";
tickets_left--;
sleep(1); // 模拟售卖时间
} else {
std::cout << "Window " << window_id << ": No more tickets left\n";
break;
}
}
unlock(lock); // 释放锁
}
int main() {
std::vector<std::thread> threads = {std::thread(sell_ticket, 1),
std::thread(sell_ticket, 2),
std::thread(sell_ticket, 3)};
for (auto& thread : threads) {
thread.join(); // 等待所有子线程完成
}
std::cout << "All tickets sold!\n";
return 0;
}
```
在这个程序中,我们创建了一个互斥锁`ticket_mutex`,然后每个售票窗口都有自己的函数`sell_ticket`。在循环中,每个窗口尝试锁定锁(`lock_guard`自动在离开作用域时解锁),如果能获取到锁并且还有票,就卖出一张票并暂停1秒。当所有票卖完或者某个窗口卖光票后,它会离开循环,并通知主线程所有票已售完。
注意,`sleep(1)`函数会阻塞当前线程1秒钟,这只是一个简化的模拟,实际应用中可能会使用更准确的计时库或异步处理来模拟购票时间。
阅读全文
相关推荐
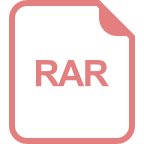
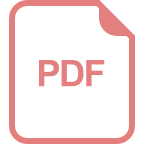
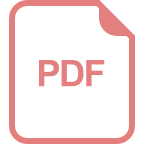













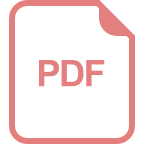
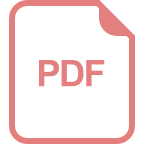
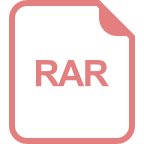