public String getJiami(@RequestBody String datas) {能有两个参数吗
时间: 2024-10-24 09:08:47 浏览: 30
在Java的HTTP控制器方法中,`@RequestBody`注解通常用于表示请求体中的数据应该作为方法参数接收。例如,你在`getJiami`方法上标注了`@RequestBody String datas`,这表明这个方法期望接收到一个名为`datas`的字符串,该字符串包含了从客户端发送过来的JSON数据。
在这个例子中,`String datas`是一个参数,它是一个单一的数据输入。如果需要两个参数,你可以添加另一个参数,但是不能直接在`@RequestBody`前加上额外的参数注解,因为`@RequestBody`本身就是一个特殊的处理方式,用于解析请求体。如果你想接收第二个独立的参数,可以考虑:
```java
public ResponseEntity<String> getJiami(@RequestBody String dataOne, String dataTwo) {
//...
}
```
这里假设`dataTwo`不是来自请求体,而是作为一个路径变量、查询参数或是其他形式传递的。如果你需要同时处理请求体和路径变量,可以考虑分开处理。请注意,每个方法的参数列表应当清晰明确,避免混淆。
相关问题
pyinstaller spec参数datas有多个文件时,数据结构是怎样的
当你使用PyInstaller工具打包Python应用并指定`spec`文件中的`datas`参数来包含多个数据文件时,数据结构通常是这样的:
在`spec`文件中,`datas`是一个列表,每个元素表示一个需要包含的数据文件对,通常包含两个部分:
1. **路径**:这是你要打包的源数据文件的实际路径,它可以是相对路径也可以是绝对路径。例如:
```python
datas = [
('relative/path/to/data1', '.'), # 将data1放入包内
('absolute/path/to/data2', 'destination/folder'), # 将data2放在destination folder下
]
```
2. **目标路径**:对于每个源文件,你可以指定在生成的应用包内的位置(如果省略,默认是当前目录)。` '.' 表示包的根目录`,`'destination/folder'`是目标文件夹。
多个数据文件会分别按照这种方式打包到指定的位置。PyInstaller会在打包后的应用程序中查找这些数据,并在运行时提供给应用程序。
std::vector<std::string> m_datas;结构含义
这个结构是在C++中声明了一个名为`m_datas`的成员变量,它的类型是`std::vector<std::string>`。`std::vector`是C++标准库中的容器类之一,用于存储多个元素的动态数组。`std::string`是表示字符串的数据类型。
在这个例子中,`m_datas`是一个存储字符串元素的向量。可以将它看作一个动态大小的字符串数组。通过使用`std::vector`,我们可以方便地添加、删除和访问其中的元素,而不需要手动管理内存。
该成员变量的完整声明是`std::vector<std::string> m_datas;`。其中,`std::vector<std::string>`表示`m_datas`是一个存储字符串元素的向量,而`m_datas`是成员变量的名称。
总结起来,`std::vector<std::string> m_datas;`的结构含义是在C++中声明了一个名为`m_datas`的成员变量,它是一个存储字符串元素的向量。
阅读全文
相关推荐
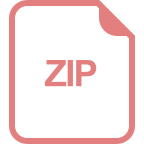
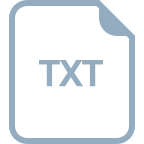
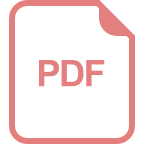













