java矩阵乘法函数
时间: 2023-11-19 20:04:45 浏览: 156
Java中可以使用如下函数实现矩阵乘法:
```
public static int[][] matrixMultiplication(int[][] a, int[][] b) {
int m = a.length;
int n = b[0].length;
int[][] result = new int[m][n];
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
for (int k = 0; k < a[0].length; k++) {
result[i][j] += a[i][k] * b[k][j];
}
}
}
return result;
}
```
此函数接收两个二维数组参数a和b,分别表示两个矩阵,返回一个新的二维数组作为结果。矩阵乘法的原理是通过对应元素的乘积相加得到新矩阵的元素。上述函数使用三个嵌套的循环来实现矩阵乘法。首先,通过变量m和n获取矩阵a和b的行数和列数。然后,创建一个新的二维数组result用于存储结果。接下来,使用三个嵌套的循环遍历矩阵a的行和矩阵b的列,并通过k变量遍历矩阵a的列或矩阵b的行,将对应元素的乘积累加到结果数组result中的对应位置。最后,返回结果数组result。
相关问题
使用Java编写一个多线程稀疏矩阵乘法运算函数
以下是使用Java编写多线程稀疏矩阵乘法运算函数的示例代码:
```java
public class SparseMatrixMultiplication {
private static final int NUM_THREADS = 4;
public static int[][] multiply(int[][] A, int[][] B) {
int m = A.length;
int n = B[0].length;
int[][] result = new int[m][n];
List<Thread> threads = new ArrayList<>();
// Divide the rows of A into NUM_THREADS parts and create a thread for each part
int chunkSize = m / NUM_THREADS;
for (int i = 0; i < NUM_THREADS; i++) {
int startRow = i * chunkSize;
int endRow = i == NUM_THREADS - 1 ? m : (i + 1) * chunkSize;
Thread thread = new Thread(new MultiplicationTask(A, B, result, startRow, endRow));
threads.add(thread);
thread.start();
}
// Wait for all threads to finish
for (Thread thread : threads) {
try {
thread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
return result;
}
private static class MultiplicationTask implements Runnable {
private int[][] A;
private int[][] B;
private int[][] result;
private int startRow;
private int endRow;
public MultiplicationTask(int[][] A, int[][] B, int[][] result, int startRow, int endRow) {
this.A = A;
this.B = B;
this.result = result;
this.startRow = startRow;
this.endRow = endRow;
}
@Override
public void run() {
int n = A[0].length;
int p = B.length;
for (int i = startRow; i < endRow; i++) {
for (int k = 0; k < n; k++) {
if (A[i][k] != 0) {
for (int j = 0; j < p; j++) {
if (B[k][j] != 0) {
result[i][j] += A[i][k] * B[k][j];
}
}
}
}
}
}
}
}
```
在此示例代码中,我们定义了一个 `SparseMatrixMultiplication` 类,其中包含一个名为 `multiply` 的函数,它接受两个稀疏矩阵 `A` 和 `B` 作为输入,并返回它们的乘积矩阵。
在函数中,我们首先创建一个大小为 `NUM_THREADS` 的线程列表,并将矩阵 `A` 的行分成 `NUM_THREADS` 个部分,为每个部分创建一个线程。每个线程都执行名为 `MultiplicationTask` 的任务,该任务将计算矩阵 `A` 的一部分与矩阵 `B` 相乘,并将结果存储在乘积矩阵 `result` 中。
`MultiplicationTask` 任务实现了 `Runnable` 接口,它接受矩阵 `A`、矩阵 `B`、乘积矩阵 `result`、开始行和结束行作为输入,并计算 `A` 的一部分与 `B` 的乘积并将结果存储在 `result` 中。
在任务中,我们首先获取矩阵 `A` 的列数 `n` 和矩阵 `B` 的行数 `p`,然后在每个行 `i` 和列 `j` 上循环乘以 `A[i][k]` 和 `B[k][j]`,其中 `k` 表示矩阵 `A` 和矩阵 `B` 的交叉点。注意,我们只计算非零元素,因为稀疏矩阵中大多数元素都是零,因此计算零元素是浪费时间和资源的。
最后,我们等待所有线程完成,并返回乘积矩阵 `result`。
Java多维数组矩阵乘法代码
在Java中,矩阵乘法通常涉及到两个二维数组(可以视为矩阵),并且它们的列数必须相等才能进行运算。这里是一个简单的例子,展示如何使用嵌套循环实现两个矩阵的乘法:
```java
public class MatrixMultiplication {
public static int[][] matrixMultiply(int[][] firstMatrix, int[][] secondMatrix) {
int rowsFirst = firstMatrix.length;
int colsFirst = firstMatrix[0].length;
int colsSecond = secondMatrix[0].length;
// 检查能否进行矩阵乘法(列数是否相等)
if (colsFirst != secondMatrix.length) {
throw new IllegalArgumentException("The number of columns in the first matrix must be equal to the number of rows in the second matrix.");
}
int resultRows = rowsFirst;
int resultCols = colsSecond;
int[][] result = new int[resultRows][resultCols];
for (int i = 0; i < resultRows; i++) {
for (int j = 0; j < resultCols; j++) {
for (int k = 0; k < colsFirst; k++) { // 计算结果矩阵的每个元素
result[i][j] += firstMatrix[i][k] * secondMatrix[k][j];
}
}
}
return result;
}
// 示例用法
public static void main(String[] args) {
int[][] a = {{1, 2}, {3, 4}};
int[][] b = {{5, 6}, {7, 8}};
int[][] c = matrixMultiply(a, b);
for (int[] row : c) {
System.out.println(Arrays.toString(row));
}
}
}
```
在这个例子中,`matrixMultiply`函数接收两个矩阵,然后通过三层循环计算出新的矩阵,并返回结果。
阅读全文
相关推荐

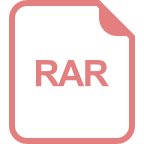



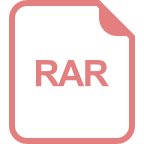







