设计一个Mapping 用来存储,账户余额。设计一个转账函数,从一个人的账户转账到另外一个人的账户。 再设计一个函数,要求可以查询任意账户的余额
时间: 2024-09-11 15:17:02 浏览: 66
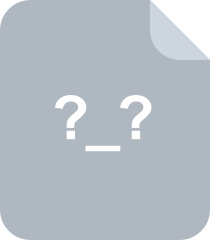
一个端口映射工具(portmapping)
在设计一个用于存储账户余额的Mapping和相关功能函数时,我们可以使用一种编程语言比如Java或Python来实现。以下是一个简单的示例:
首先,我们设计一个简单的账户类,包含账户ID和余额属性:
```java
public class Account {
private String accountId;
private double balance;
public Account(String accountId) {
this.accountId = accountId;
this.balance = 0.0;
}
public String getAccountId() {
return accountId;
}
public double getBalance() {
return balance;
}
public void setBalance(double balance) {
this.balance = balance;
}
}
```
接下来,我们设计一个转账函数,用于从一个账户转账到另一个账户:
```java
public class BankingSystem {
private Map<String, Account> accounts = new HashMap<>();
public void addAccount(Account account) {
accounts.put(account.getAccountId(), account);
}
public void transfer(String fromAccountId, String toAccountId, double amount) {
Account fromAccount = accounts.get(fromAccountId);
Account toAccount = accounts.get(toAccountId);
if (fromAccount == null || toAccount == null) {
throw new IllegalArgumentException("账户不存在");
}
if (fromAccount.getBalance() < amount) {
throw new IllegalArgumentException("余额不足");
}
fromAccount.setBalance(fromAccount.getBalance() - amount);
toAccount.setBalance(toAccount.getBalance() + amount);
}
public double getAccountBalance(String accountId) {
Account account = accounts.get(accountId);
if (account == null) {
throw new IllegalArgumentException("账户不存在");
}
return account.getBalance();
}
}
```
在这个类中,`accounts`是一个存储`Account`对象的`Map`。我们使用`addAccount`方法来添加账户,使用`transfer`方法来进行转账操作,使用`getAccountBalance`方法来查询账户余额。
需要注意的是,在实际应用中,转账操作应该是线程安全的,并且要处理并发问题,确保在多线程环境下数据的一致性和完整性。此外,还需要进行错误处理和输入验证等。
阅读全文
相关推荐
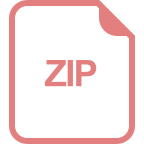
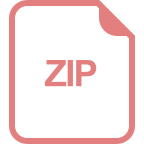
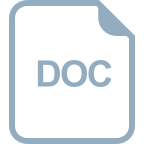
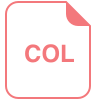
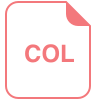
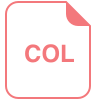
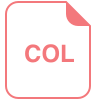
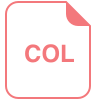
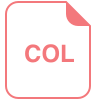




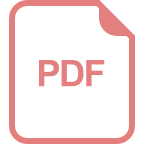
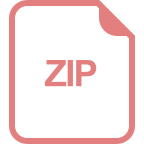
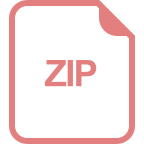
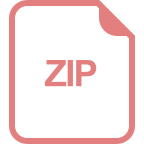