java NP算法样例
时间: 2023-11-10 12:50:29 浏览: 94
Sure! Here's an example of the NP algorithm implemented in Java:
```java
import java.util.ArrayList;
import java.util.Arrays;
public class NpAlgorithmExample {
public static boolean npAlgorithm(int[] set, int targetSum) {
// Sort the set in non-decreasing order
Arrays.sort(set);
// Initialize the sum variable
int currentSum = 0;
// Create an empty list to store the subset
ArrayList<Integer> subset = new ArrayList<>();
// Call the recursive helper function to find the subset
return findSubset(set, targetSum, 0, currentSum, subset);
}
private static boolean findSubset(int[] set, int targetSum, int index, int currentSum, ArrayList<Integer> subset) {
// Base cases
if (currentSum == targetSum) {
// Print the subset
System.out.println("Subset found: " + subset);
return true;
}
if (currentSum > targetSum || index >= set.length) {
return false;
}
// Recursive cases
for (int i = index; i < set.length; i++) {
// Add the current element to the subset
subset.add(set[i]);
// Recursive call with the next index and updated current sum
if (findSubset(set, targetSum, i + 1, currentSum + set[i], subset)) {
return true;
}
// Remove the current element from the subset
subset.remove(subset.size() - 1);
}
return false;
}
public static void main(String[] args) {
int[] set = {1, 2, 3, 4, 5};
int targetSum = 7;
System.out.println("Set: " + Arrays.toString(set));
System.out.println("Target Sum: " + targetSum);
boolean subsetFound = npAlgorithm(set, targetSum);
if (!subsetFound) {
System.out.println("No subset found!");
}
}
}
```
In this example, the NP algorithm is used to find a subset of a given set of numbers whose sum is equal to a target sum. The algorithm uses a recursive approach to iterate through all possible combinations of elements in the set. The `npAlgorithm` method is the entry point which initializes the necessary variables and calls the recursive helper function `findSubset`. The `findSubset` function checks for base cases, such as finding the subset with the target sum or exceeding the target sum, and performs recursive calls to explore different combinations of elements. Finally, the `main` method demonstrates how to use the algorithm by providing a sample set and target sum.
阅读全文
相关推荐
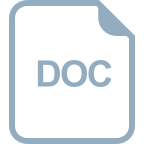
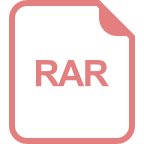
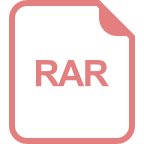
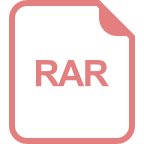
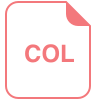
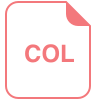
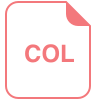
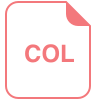
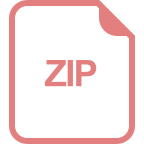
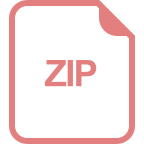
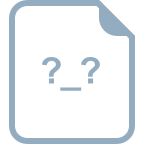
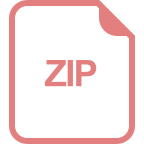
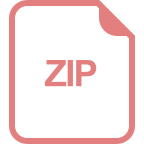
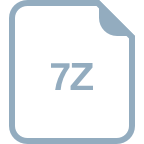
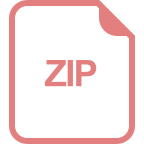