运用C语言,分别通过头插法,尾插法建立字符链表,要求至少a~e五个元素,通过头指针挨个输入元素并最终销毁。并写出主函数分别实现
时间: 2024-09-18 10:16:51 浏览: 52
在C语言中,我们可以使用结构体来表示链表节点,并分别用头插法(Prepend)和尾插法(Append)来创建链表。这里我会给出基本的代码示例,包括如何插入元素、遍历以及销毁链表。
首先,定义链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
char data;
struct Node* next;
} Node;
// 头插法添加节点
Node* prepend(Node** head, char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = *head;
*head = newNode;
return *head;
}
// 尾插法添加节点
Node* append(Node** head, char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (*head == NULL) {
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
newNode->data = data;
newNode->next = NULL; // 初始化新节点的下一个为空
return *head;
}
// 主函数,用于创建链表、输入数据和销毁链表
void createAndDestroy(char ch, Node** head) {
// 创建链表
for (char c = 'a'; c <= ch; c++) {
switch (c) {
case 'a':
*head = prepend(head, c);
break;
default:
*head = append(head, c);
break;
}
}
// 遍历链表并打印
Node* temp = *head;
while (temp != NULL) {
printf("%c ", temp->data);
temp = temp->next;
}
printf("\n");
// 销毁链表
while (*head != NULL) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
}
printf("链表已销毁。\n");
}
int main() {
Node* head = NULL;
createAndDestroy('e', &head); // 使用'e'作为结束元素
return 0;
}
```
在这个示例中,`createAndDestroy` 函数会按照指定的字符范围(这里是'a'到'e'),先用头插法初始化链表,然后使用尾插法添加剩余元素。最后,它会遍历并打印链表,然后逐个删除节点并释放内存。在`main`函数中调用这个函数即可完成整个过程。
阅读全文
相关推荐
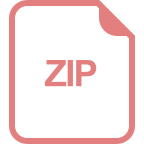
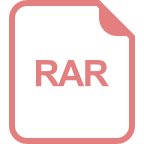
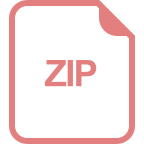

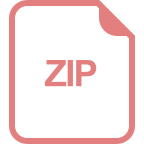
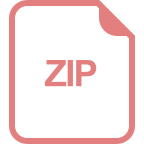
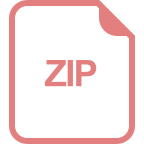
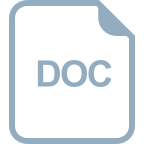
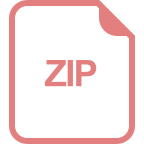
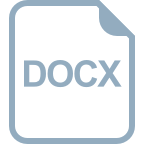
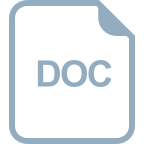
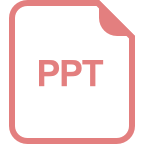
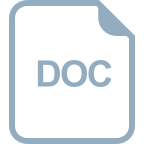
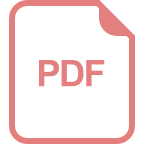
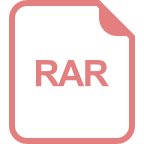