泳C语言设计一个容纳二维向量的结构,设计用于初始化、加法、减法、标量乘法、长度、点积的函数,设计至少3项功能
时间: 2024-09-23 13:04:57 浏览: 34
在C语言中,我们可以设计一个名为`Vector2D`的结构体,它包含两个浮点数元素(通常是x和y坐标),并提供相应的操作方法。以下是结构体定义以及初始化、加法、减法、标量乘法、长度和点积等函数的实现:
```c
#include <stdio.h>
#include <math.h>
// 定义二维向量结构体
typedef struct {
float x;
float y;
} Vector2D;
// 初始化函数
Vector2D init_vector(float x, float y) {
Vector2D vec;
vec.x = x;
vec.y = y;
return vec;
}
// 加法函数
Vector2D add(Vector2D v1, Vector2D v2) {
Vector2D result;
result.x = v1.x + v2.x;
result.y = v1.y + v2.y;
return result;
}
// 减法函数
Vector2D subtract(Vector2D v1, Vector2D v2) {
Vector2D result;
result.x = v1.x - v2.x;
result.y = v1.y - v2.y;
return result;
}
// 标量乘法函数
Vector2D scale(Vector2D v, float scalar) {
Vector2D scaled_vec;
scaled_vec.x = v.x * scalar;
scaled_vec.y = v.y * scalar;
return scaled_vec;
}
// 长度(模)函数
float length(Vector2D v) {
return sqrt(v.x * v.x + v.y * v.y);
}
// 点积函数
float dot_product(Vector2D v1, Vector2D v2) {
return v1.x * v2.x + v1.y * v2.y;
}
int main() {
Vector2D v1 = init_vector(1.0f, 2.0f);
Vector2D v2 = init_vector(3.0f, 4.0f);
printf("v1: (%f, %f)\n", v1.x, v1.y);
printf("v2: (%f, %f)\n", v2.x, v2.y);
Vector2D sum = add(v1, v2);
Vector2D diff = subtract(v1, v2);
Vector2D scaled_v = scale(v1, 2.5);
printf("Addition: (%f, %f)\n", sum.x, sum.y);
printf("Subtraction: (%f, %f)\n", diff.x, diff.y);
printf("Scaling (2.5): (%f, %f)\n", scaled_v.x, scaled_v.y);
float len_v1 = length(v1);
float dp = dot_product(v1, v2);
printf("Length of v1: %.2f\n", len_v1);
printf("Dot product: %.2f\n", dp);
return 0;
}
```
阅读全文
相关推荐
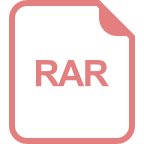
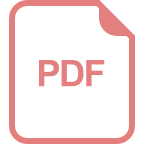
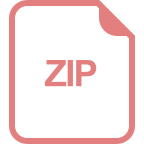















