currentHashMap 使用案例
时间: 2023-08-28 22:18:03 浏览: 94
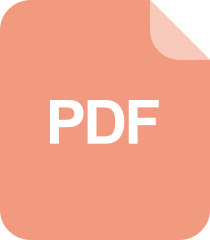
JS hashMap实例详解
ConcurrentHashMap 是 HashMap 的线程安全版本,适用于多线程环境。下面是一些 ConcurrentHashMap 的使用案例:
1. 并发读写操作:多个线程同时读写 ConcurrentHashMap。它可以安全地处理并发读写操作,而无需额外的同步机制。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
map.put("key2", 2);
// 并发读取
int value1 = map.get("key1");
int value2 = map.get("key2");
// 并发写入
map.put("key3", 3);
```
2. 迭代操作:使用迭代器遍历 ConcurrentHashMap 中的键值对。它提供弱一致性保证,即迭代期间其他线程对该 ConcurrentHashMap 的更新可能不可见。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
map.put("key2", 2);
Iterator<Map.Entry<String, Integer>> iterator = map.entrySet().iterator();
while (iterator.hasNext()) {
Map.Entry<String, Integer> entry = iterator.next();
String key = entry.getKey();
int value = entry.getValue();
System.out.println(key + ": " + value);
}
```
3. 原子操作:使用原子操作方法更新 ConcurrentHashMap 中的值。ConcurrentHashMap 提供了一些原子操作方法,如 `putIfAbsent()`、`replace()`、`computeIfAbsent()` 等。
```java
ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
map.put("key1", 1);
// 原子更新
int newValue = map.computeIfAbsent("key1", key -> key.length());
System.out.println("New value: " + newValue);
```
这些只是 ConcurrentHashMap 的一些使用案例,它还提供了其他丰富的功能,如批量操作、并发控制等。
阅读全文
相关推荐
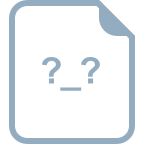

















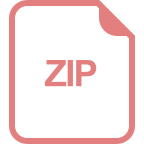