用c语言编写最小二乘法
时间: 2024-12-06 22:22:42 浏览: 23
在C语言中,最小二乘法是一种常用的数据拟合方法,用于通过找到一条直线或曲线来近似数据点,使得所有数据点到这条线的垂直距离之和(残差平方和)最小。以下是一个简单的二维直线最小二乘法求解的例子:
```c
#include <stdio.h>
#include <math.h>
// 计算斜率和截距
void linear_regression(float x[], float y[], int n, float *slope, float *intercept) {
float sum_x = 0, sum_y = 0, sum_xy = 0, sum_xx = 0;
for (int i = 0; i < n; ++i) {
sum_x += x[i];
sum_y += y[i];
sum_xy += x[i] * y[i];
sum_xx += x[i] * x[i];
}
*slope = (n * sum_xy - sum_x * sum_y) / (n * sum_xx - sum_x * sum_x);
*intercept = (sum_y - (*slope) * sum_x) / n;
}
int main() {
float x[] = {1, 2, 3, 4, 5};
float y[] = {2, 4, 5, 4, 5}; // 示例数据点
int n = sizeof(x) / sizeof(x[0]);
float slope, intercept;
linear_regression(x, y, n, &slope, &intercept);
printf("y = %.2fx + %.2f\n", slope, intercept); // 输出最佳拟合线方程
return 0;
}
```
这个程序首先计算x和y的总和以及xy和xx的总积,然后利用公式计算斜率和截距。在`main()`函数中,我们提供一组示例数据,并调用`linear_regression`函数求得最接近这些数据的直线。
阅读全文
相关推荐




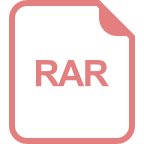













