线性表的插入与删除的c++代码
时间: 2024-09-23 16:05:04 浏览: 39
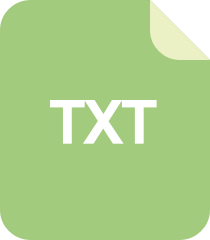
线性表(c++代码)
线性表在C++中通常可以使用数组或链表这两种数据结构来实现,这里分别给出插入和删除操作的例子:
### 数组实现(动态数组,如vector)
```cpp
#include <iostream>
#include <vector>
// 使用vector,它是动态数组,内部处理了扩容和收缩
void insertVector(std::vector<int>& list, int value) {
list.push_back(value);
}
void removeElementVector(std::vector<int>& list, int target) {
auto it = std::find(list.begin(), list.end(), target);
if (it != list.end()) {
list.erase(it);
}
}
int main() {
std::vector<int> numbers;
insertVector(numbers, 5); // 插入元素5
removeElementVector(numbers, 5); // 删除元素5
for (const auto& num : numbers) {
std::cout << num << " ";
}
return 0;
}
```
### 链表实现(单向链表)
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkedList {
private:
Node* head;
public:
void insert(int value) {
Node* newNode = new Node{value, nullptr};
if (!head) {
head = newNode;
} else {
Node* temp = head;
while (temp->next) {
temp = temp->next;
}
temp->next = newNode;
}
}
void remove(int value) {
if (!head) return;
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
while (current->next && current->next->data != value) {
current = current->next;
}
if (current->next) {
current->next = current->next->next;
delete current->next;
}
}
};
int main() {
LinkedList list;
list.insert(5); // 插入元素5
list.remove(5); // 删除元素5
return 0;
}
```
阅读全文
相关推荐
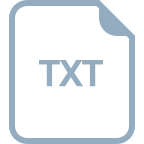
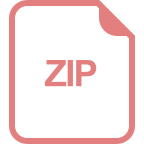















