设在一个房间内,有一个机器人,一个壁橱,一个积木块,两张桌子A和B。机器人在壁橱的旁边,且两手空空。桌子A上放着积木块,桌子B上是空的。机器然后回到壁橱边。请用完整的python人把积木块从桌子A上转移到桌子B上,代码来表示机器人转移积木块的过程
时间: 2024-09-19 22:02:05 浏览: 47
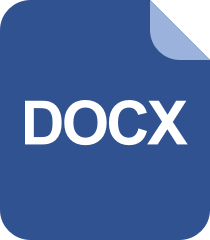
FANUC机器人将两个程序中的示教定位点设置成同一个坐标点的处理方法.docx

在这个场景中,我们可以用Python来模拟这个过程,假设我们的机器人有基本的操作能力,比如移动和拿起物品。这里是一个简单的示例:
```python
class Robot:
def __init__(self):
self.location = "壁橱旁边"
self.holding = []
# 移动到某个位置
def move(self, destination):
if destination == "桌子A":
print("机器人正在移动到桌子A")
elif destination == "桌子B":
print("机器人正在移动到桌子B")
else:
print(f"机器人无法移动到{destination}")
# 拿起物品
def pick_up(self, item):
if item == "积木块":
if not self.holding:
self.holding.append(item)
print("机器人拿起积木块")
else:
print("机器人手里已经有东西,无法再次拾取")
else:
print(f"机器人无法拿起{item}")
# 放下物品
def put_down(self, item):
if item in self.holding:
self.holding.remove(item)
if len(self.holding) == 0:
print("机器人放下积木块")
else:
print("机器人放下物品")
else:
print(f"机器人手中没有{item},无法放下")
# 创建机器人实例
robot = Robot()
# 机器人移动到桌子A并拿起积木块
robot.move("桌子A")
robot.pick_up("积木块")
# 机器人移动到桌子B并放下积木块
robot.move("桌子B")
robot.put_down("积木块")
```
注意,这只是一个基础的模拟,现实情况可能需要更复杂的状态管理和交互模型。
阅读全文
相关推荐
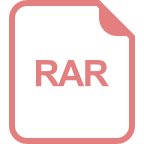
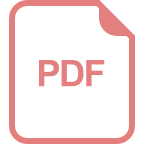






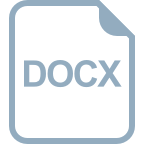
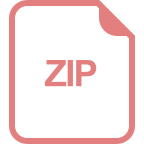
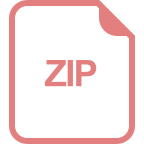
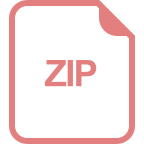
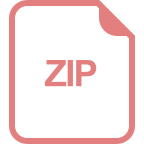
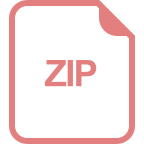