c语言获取本机cpuid及mac地址
时间: 2023-07-30 18:00:56 浏览: 80
C语言可以通过使用Windows和Linux操作系统提供的相关 API 来获取本机的 CPUID 和 MAC 地址。
首先,我们可以通过Windows API函数`GetSystemInfo()`获取CPUID。示例代码如下:
```c
#include <stdio.h>
#include <windows.h>
void main()
{
SYSTEM_INFO sys_info;
GetSystemInfo(&sys_info);
printf("CPU ID: %x %x %x %x\n", sys_info.dwProcessorType, sys_info.dwPageSize, sys_info.dwActiveProcessorMask, sys_info.dwNumberOfProcessors);
}
```
然后,我们可以通过使用Windows API函数`GetAdaptersInfo()`来获取本机的 MAC 地址。示例代码如下:
```c
#include <stdio.h>
#include <windows.h>
#include <iphlpapi.h>
#pragma comment(lib, "iphlpapi.lib")
void main()
{
IP_ADAPTER_INFO adapter_info[16];
DWORD buf_len = sizeof(adapter_info);
if (GetAdaptersInfo(adapter_info, &buf_len) == ERROR_SUCCESS)
{
PIP_ADAPTER_INFO curr_adapter = adapter_info;
while (curr_adapter != NULL)
{
printf("MAC Address: %02x:%02x:%02x:%02x:%02x:%02x\n",
curr_adapter->Address[0], curr_adapter->Address[1], curr_adapter->Address[2],
curr_adapter->Address[3], curr_adapter->Address[4], curr_adapter->Address[5]);
curr_adapter = curr_adapter->Next;
}
}
}
```
对于Linux操作系统,我们可以使用`sys/sysinfo.h`和`ifaddrs.h`头文件来获取 CPUID 和 MAC 地址。示例代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/sysinfo.h>
#include <ifaddrs.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <string.h>
void main()
{
struct sysinfo sys_info;
if (sysinfo(&sys_info) == 0)
{
printf("CPU ID: %08x\n", (unsigned int)sys_info.processor_id[0]);
}
struct ifaddrs* ifaddr, *ifa;
if (getifaddrs(&ifaddr) == 0)
{
for (ifa = ifaddr; ifa != NULL; ifa = ifa->ifa_next)
{
if (ifa->ifa_addr == NULL || ifa->ifa_addr->sa_family != AF_PACKET)
continue;
struct sockaddr_ll* s = (struct sockaddr_ll*)ifa->ifa_addr;
unsigned char mac[6];
memcpy(mac, s->sll_addr, 6);
printf("MAC Address: %02x:%02x:%02x:%02x:%02x:%02x\n",
mac[0], mac[1], mac[2], mac[3], mac[4], mac[5]);
}
freeifaddrs(ifaddr);
}
}
```
需要注意的是,在使用这些API函数时,可能需要添加某些特定的库文件或在编译选项中指定相关的库文件,具体需要根据不同的操作系统和编译环境来进行调整。
相关推荐
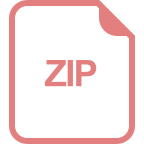














