用C语言编写创建2个线程的程序。父进程显示5次“This is the parent , pid is xxxxxx”, 每个线程显示5次“This is a new thread ,thread ID is xxxxxx”。要求交叉显示父进程和子进程的显示内容。
时间: 2024-10-27 12:08:16 浏览: 118
在C语言中,我们可以使用`pthread.h`库来创建和管理线程。以下是一个简单的示例程序,展示了如何创建两个线程,父进程显示消息并等待子线程完成:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define MAX_MSG 5
void* print_thread(void* arg) {
int tid = (int)arg;
char msg[64] = "This is a new thread, thread ID is %d\n";
for (int i = 0; i < MAX_MSG; i++) {
sprintf(msg, msg, tid);
printf("%s", msg);
// 线程自行控制睡眠时间以交错输出
sleep(1); // 这里假设我们有休眠函数,实际应用中可能需要替换为其他同步机制
}
pthread_exit(NULL);
}
int main() {
int parent_pid = getpid();
printf("Parent process started, PID: %d\n", parent_pid);
pthread_t thread_id1, thread_id2;
if (pthread_create(&thread_id1, NULL, print_thread, (void*)1)) {
perror("Error creating thread 1");
return 1;
}
if (pthread_create(&thread_id2, NULL, print_thread, (void*)2)) {
perror("Error creating thread 2");
return 1;
}
// 主线程等待子线程结束
pthread_join(thread_id1, NULL);
pthread_join(thread_id2, NULL);
printf("Both threads finished.\n");
return 0;
}
```
在这个程序中,我们首先在主进程中打印一条消息,并获取父进程ID。然后,我们创建两个线程,每个线程都有一个唯一的标识符作为参数传递给`print_thread`函数。这个函数内部,我们会循环显示5次指定的消息,并在每次之间短暂休眠,以实现交错显示。
阅读全文
相关推荐
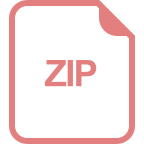
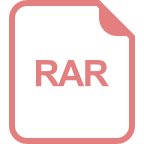
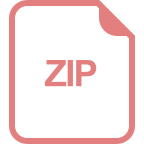















