帮我用c语言写一个多进程或多线程程序
时间: 2024-09-23 21:09:18 浏览: 47
在C语言中,我们可以使用POSIX线程库(pthreads)来创建多线程程序,或者使用`fork()`函数创建多进程。这里是一个简单的多线程示例:
```c
#include <stdio.h>
#include <pthread.h>
// 创建线程的函数
void *thread_function(void *arg) {
printf("This is thread %lu, running...\n", (long unsigned int)arg);
return NULL;
}
int main() {
pthread_t thread_id; // 线程ID
int rc;
// 创建新线程
if ((rc = pthread_create(&thread_id, NULL, thread_function, (void *)1)) != 0) {
fprintf(stderr, "Error creating thread: %d\n", rc);
exit(-1);
}
// 主线程继续执行
printf("Main thread is waiting...\n");
pthread_join(thread_id, NULL); // 等待新线程结束
printf("Thread %lu has finished.\n", (long unsigned int)1);
return 0;
}
```
而对于多进程,可以参考下面的例子:
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
int main() {
pid_t child_pid; // 子进程ID
int status;
// fork()创建新的进程
if ((child_pid = fork()) == 0) { // 如果是子进程
printf("Child process started with PID: %d\n", getpid());
sleep(2); // 模拟工作
_exit(0); // 结束子进程
} else if (child_pid > 0) { // 父进程
printf("Parent process started, waiting for child PID: %d\n", child_pid);
waitpid(child_pid, &status, 0); // 等待子进程结束
printf("Child process ended with status: %d\n", status);
} else { // fork()失败
perror("Fork failed");
exit(EXIT_FAILURE);
}
return 0;
}
```
阅读全文
相关推荐
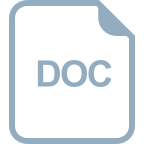
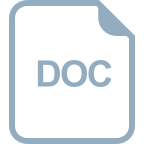
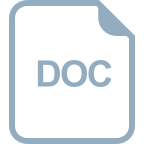
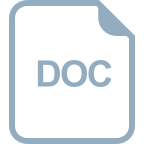
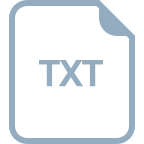
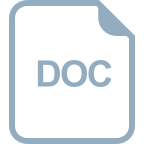
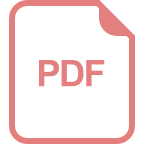
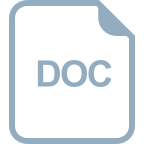
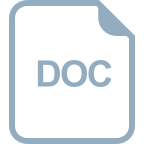

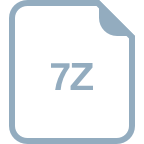
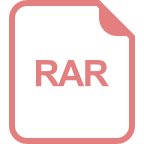
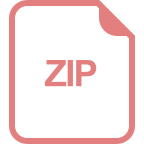
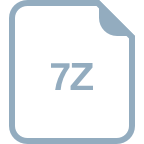
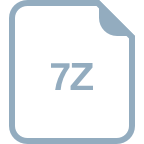
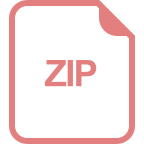