实现 strStr() 函数。 给你两个字符串haystack和needle,请你在haystack字符串中找出needle字符串出现的第一个位置(下标从0开始)。如果不存在,则返回-1。完整代码
时间: 2024-10-01 19:02:01 浏览: 44
`strStr()`函数是一个常见的查找子串的问题,通常用于寻找在一个长字符串(haystack)中第一次出现特定子字符串(needle)的位置。这个函数在许多编程语言中都有相应的实现。下面是一个简单的Python版本的例子,它使用了双指针法:
```python
def strStr(haystack, needle):
if not needle:
return 0
haystack_len = len(haystack)
needle_len = len(needle)
# 搜索从头开始
for i in range(haystack_len - needle_len + 1):
# 如果子串匹配,返回起始索引
if haystack[i:i+needle_len] == needle:
return i
# 如果没有找到,返回-1
return -1
```
在这个函数中,我们首先检查needle是否为空,因为空字符串会在任何地方都存在。然后,我们初始化两个指针,一个指向haystack的开头,另一个指向needle的开头。我们会逐字符比较,如果找到匹配的部分,就更新结果并继续搜索;如果没有找到匹配,我们就向右移动haystack的指针。当haystack遍历完还没找到匹配,说明needle不在haystack中,返回-1。
相关问题
用java代码写出给你两个字符串 haystack 和 needle ,请你在 haystack 字符串中找出 needle 字符串的第一个匹配项的下标(下标从 0 开始)。如果 needle 不是 haystack 的一部分,则返回 -1 。
### 回答1:
可以的,以下是Java代码实现:
```
public int strStr(String haystack, String needle) {
if (needle.isEmpty()) {
return 0;
}
int hLen = haystack.length();
int nLen = needle.length();
if (hLen < nLen) {
return -1;
}
for (int i = 0; i <= hLen - nLen; i++) {
if (haystack.substring(i, i + nLen).equals(needle)) {
return i;
}
}
return -1;
}
```
这个函数接收两个字符串参数haystack和needle,返回needle在haystack中第一次出现的位置,如果没有匹配项,则返回-1。函数使用substring()函数来比较haystack中的每个子字符串是否与needle相等,从而实现字符串匹配。
### 回答2:
可以使用Java中的字符串方法来实现题目要求。以下是一种可能的解决方案:
```java
public class Solution {
public int strStr(String haystack, String needle) {
if (needle.length() == 0) {
return 0;
}
for (int i = 0; i <= haystack.length() - needle.length(); i++) {
if (haystack.substring(i, i + needle.length()).equals(needle)) {
return i;
}
}
return -1;
}
}
```
以上代码中,我们首先判断 needle 是否为空字符串,如果是,则直接返回 0,因为空字符串一定是 haystack 的子串。然后,我们使用 for 循环遍历从 0 到 haystack.length() - needle.length() 的下标范围。在循环过程中,我们使用 haystack.substring(i, i + needle.length()) 获取长度与 needle 相等的子串,并将其与 needle 进行比较。如果相等,则返回当前下标 i,表示找到了子串的第一个匹配项。如果循环结束后仍未找到匹配项,则说明 needle 不是 haystack 的子串,返回 -1。
以上就是根据题目要求用 Java 代码编写的解决方案。
### 回答3:
可以使用Java代码来实现在haystack字符串中找出needle字符串的第一个匹配项的下标,代码如下所示:
```java
public class FindIndex {
public static int findIndex(String haystack, String needle) {
if (haystack == null || needle == null || needle.length() > haystack.length()) {
return -1;
}
int haystackLen = haystack.length();
int needleLen = needle.length();
for (int i = 0; i <= haystackLen - needleLen; i++) {
int j;
for (j = 0; j < needleLen; j++) {
if (haystack.charAt(i + j) != needle.charAt(j)) {
break;
}
}
if (j == needleLen) {
return i;
}
}
return -1;
}
public static void main(String[] args) {
String haystack = "hello world";
String needle = "world";
int index = findIndex(haystack, needle);
System.out.println("在haystack字符串中找到needle字符串的第一个匹配项的下标为:" + index);
}
}
```
以上代码通过双重循环遍历haystack字符串和needle字符串,逐个字符进行比较,如果找到相同的字符序列,则返回对应的下标位置。如果遍历完整个haystack字符串仍未找到匹配项,则返回-1。在上述代码中,给定的haystack字符串为"hello world",needle字符串为"world",其输出结果为:
```
在haystack字符串中找到needle字符串的第一个匹配项的下标为:6
```
表示在haystack字符串的下标为6处找到了needle字符串的第一个匹配项。
字符串操作函数strstr
`strstr` 是一个在 C 语言中用于查找一个字符串中是否包含另一个子字符串的函数。它的函数原型如下:
```c
char *strstr(const char *haystack, const char *needle);
```
其中,`haystack` 是待搜索的字符串,`needle` 是要查找的子字符串。`strstr` 函数会返回一个指针,指向 `haystack` 中第一次出现 `needle` 的位置,如果没有找到,则返回空指针。
以下是一个使用 `strstr` 函数的例子:
```c
#include <stdio.h>
#include <string.h>
int main() {
char str[] = "Hello, world!";
char *ptr = strstr(str, "world");
if (ptr != NULL) {
printf("substring found at index: %ld\n", ptr - str);
} else {
printf("substring not found\n");
}
return 0;
}
```
输出结果为:
```
substring found at index: 7
```
这个例子中,我们在字符串 `str` 中使用 `strstr` 函数查找子字符串 "world",并在找到时输出它的索引位置。
阅读全文
相关推荐
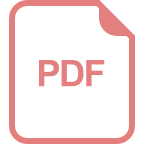
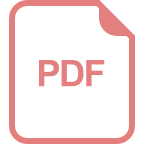
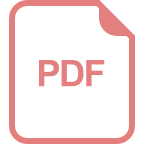
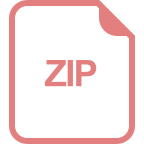
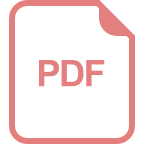
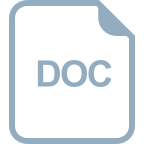
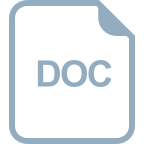






