用c++定义学生结构体,用一个函数实现学生数据的输入,一个函数计算每个学生三门课的成绩,一个函数输出平均分最高的学生的数据。
时间: 2024-12-15 16:13:09 浏览: 6
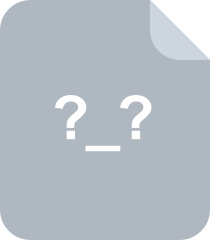
2.编写一个函数print, 打印一个学生的成绩数组, 该数组中有5个学生的数据记录,每个记录包括num,name,score[3],用主函数输入这些记录,用p
在C++中,我们可以定义一个名为`Student`的结构体来存储学生的基本信息,包括姓名、学号以及三门课程的成绩。首先,我们需要创建一个包含这些属性的结构体:
```cpp
#include <iostream>
#include <string>
using namespace std;
// 定义学生结构体
struct Student {
string name;
int id;
float score1; // 第一门课成绩
float score2; // 第二门课成绩
float score3; // 第三门课成绩
};
```
接下来,我们分别编写三个函数来实现所需的功能:
1. 输入学生数据的函数:
```cpp
void inputStudent(Student& student) {
cout << "请输入学生信息: ";
cin >> student.name >> student.id >> student.score1 >> student.score2 >> student.score3;
}
```
2. 计算每名学生总成绩的函数:
```cpp
float calculateTotalScore(const Student& student) {
return student.score1 + student.score2 + student.score3;
}
```
3. 找出平均分最高的学生的输出函数:
```cpp
void findHighestAverage(const vector<Student>& students) {
double highestAverage = 0.0;
Student highestAverageStudent;
for (const auto& student : students) {
float totalScore = calculateTotalScore(student);
double average = static_cast<double>(totalScore) / 3.0;
if (average > highestAverage) {
highestAverage = average;
highestAverageStudent = student;
}
}
cout << "平均分最高的学生是:\n";
cout << "姓名: " << highestAverageStudent.name << "\n";
cout << "学号: " << highestAverageStudent.id << "\n";
cout << "平均成绩: " << highestAverage << endl;
}
```
最后,你需要在主程序中创建一个学生向量,并使用输入函数添加学生,然后调用`findHighestAverage`函数来找出最高平均分的学生。
```cpp
int main() {
vector<Student> students;
Student currentStudent;
// 获取用户输入并创建学生对象
while (true) {
inputStudent(currentStudent);
students.push_back(currentStudent); // 添加到学生列表中
cout << "是否还要输入其他学生? (y/n): ";
char response;
cin >> response;
if (response != 'y') break; // 用户选择结束输入
}
findHighestAverage(students);
return 0;
}
```
阅读全文
相关推荐
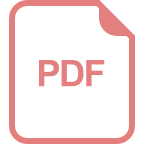
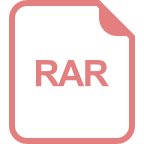















