mysql多表更新,有mapper,entity,service
时间: 2024-04-15 08:27:35 浏览: 138
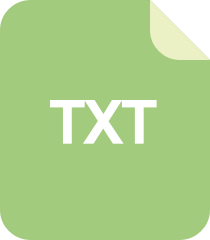
SQL多表更新
对于MySQL的多表更新,你可以通过使用Mapper、Entity和Service来实现。下面是一种常见的实现方式:
首先,在Mapper中定义多表更新的SQL语句。例如,假设你有两张表:TableA和TableB,你想要根据某个条件更新它们的数据。你可以在Mapper中定义一个更新语句,类似于下面的示例:
```java
@Mapper
public interface YourMapper {
@Update("UPDATE table_a a, table_b b SET a.column1 = #{value1}, b.column2 = #{value2} WHERE a.id = b.id AND a.condition = #{condition}")
void updateTables(@Param("value1") String value1, @Param("value2") String value2, @Param("condition") String condition);
}
```
接下来,在Entity中定义对应的表结构。你需要创建两个实体类,分别对应TableA和TableB的结构。
```java
public class TableA {
private Integer id;
private String column1;
// 其他属性及其对应的getter和setter方法
}
public class TableB {
private Integer id;
private String column2;
// 其他属性及其对应的getter和setter方法
}
```
然后,在Service中编写业务逻辑代码。你可以注入Mapper,并在Service方法中调用Mapper的更新方法。
```java
@Service
public class YourService {
private final YourMapper yourMapper;
public YourService(YourMapper yourMapper) {
this.yourMapper = yourMapper;
}
public void updateTables(String value1, String value2, String condition) {
yourMapper.updateTables(value1, value2, condition);
}
}
```
最后,你可以在需要的地方调用Service方法来进行多表更新操作。
```java
@Service
public class YourController {
private final YourService yourService;
public YourController(YourService yourService) {
this.yourService = yourService;
}
public void updateTables() {
String value1 = "new value 1";
String value2 = "new value 2";
String condition = "some condition";
yourService.updateTables(value1, value2, condition);
}
}
```
这样,你就可以使用Mapper、Entity和Service来实现MySQL的多表更新操作了。当然,具体的代码实现可能会根据你的需求和框架有所不同,以上只是一个简单的示例。
阅读全文
相关推荐
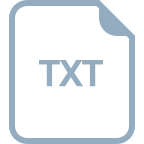
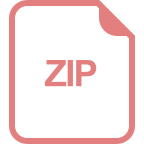
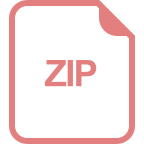










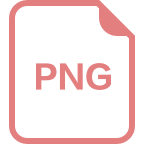
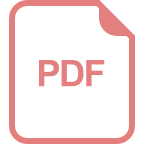