sheet.draw(graphics2D);没有draw()方法
时间: 2024-04-24 20:23:26 浏览: 35
非常抱歉,我在之前的回答中给出了错误的信息。事实上,Apache POI库没有提供直接将Excel绘制到Graphics2D对象的方法。相反,你可以使用Apache POI库提供的方法来读取Excel文件中的内容,然后使用其他图像处理库(例如Java的AWT或第三方库)将内容绘制到图像中。
下面是一个使用Apache POI和Java的AWT库将Excel内容绘制到图像中的示例代码:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.*;
import javax.imageio.ImageIO;
import java.awt.*;
import java.awt.image.BufferedImage;
import java.io.*;
public class ExcelToImageConverter {
public static void main(String[] args) {
try {
// 加载Excel文件
FileInputStream fileInputStream = new FileInputStream("path/to/excel.xlsx");
Workbook workbook = new XSSFWorkbook(fileInputStream);
Sheet sheet = workbook.getSheetAt(0);
// 获取Excel的宽度和高度
int width = sheet.getColumnWidth(sheet.getRow(0).getLastCellNum() - 1);
int height = (int) (sheet.getLastRowNum() * sheet.getDefaultRowHeight());
// 创建BufferedImage对象
BufferedImage bufferedImage = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
Graphics2D graphics2D = bufferedImage.createGraphics();
// 设置背景色
graphics2D.setColor(Color.WHITE);
graphics2D.fillRect(0, 0, width, height);
// 设置字体
Font font = new Font("Arial", Font.PLAIN, 12);
graphics2D.setFont(font);
// 绘制Excel内容到BufferedImage对象
int rowHeight = sheet.getDefaultRowHeight();
int numCols = sheet.getRow(0).getLastCellNum();
for (int i = 0; i <= sheet.getLastRowNum(); i++) {
Row row = sheet.getRow(i);
for (int j = 0; j < numCols; j++) {
Cell cell = row.getCell(j);
if (cell != null) {
String cellValue = cell.toString();
int colWidth = sheet.getColumnWidth(j);
int x = sheet.getColumnWidthInPixels(j);
int y = i * rowHeight;
graphics2D.drawString(cellValue, x, y + rowHeight);
}
}
}
// 保存BufferedImage对象为图片文件
File outputFile = new File("path/to/output.png");
ImageIO.write(bufferedImage, "png", outputFile);
// 关闭资源
graphics2D.dispose();
workbook.close();
fileInputStream.close();
System.out.println("Excel转换成图片成功!");
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
在这个示例中,我们使用了`graphics2D.drawString()`方法来将每个单元格的文本绘制到图像中。你可以根据需要调整字体、颜色和位置等绘制参数。同样,记得将`"path/to/excel.xlsx"`替换为你的Excel文件路径,将`"path/to/output.png"`替换为你想要保存的图片文件路径。
请注意,这只是一个简单的示例,处理复杂的Excel文件可能需要更多的代码逻辑。此外,在处理大型Excel文件时,可能需要进行分页处理以适应图像的尺寸限制。
相关推荐
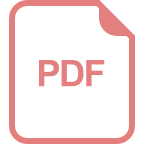
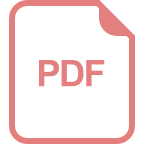
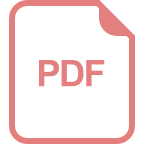







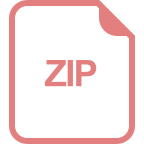
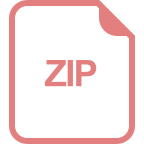