数组,函数:输入学生成绩, 进行中 求最大值、最小值、排序 c++
时间: 2024-10-21 19:07:40 浏览: 55
在C++中,你可以使用数组和函数来处理一组学生成绩,包括查找最大值、最小值并排序。下面是一个简单的例子:
```cpp
#include <iostream>
#include <algorithm> // 包含算法库以便使用sort
// 定义结构体表示学生,包含成绩属性
struct Student {
int score;
};
// 函数声明,用于计算最大值和最小值
int findMax(const std::vector<Student>& scores);
int findMin(const std::vector<Student>& scores);
// 函数实现,寻找并返回数组中的最大值和最小值
int findMax(const std::vector<Student>& scores) {
if (scores.empty()) return INT_MIN; // 如果数组为空,返回整数最小值
int maxScore = scores[0].score;
for (const auto& student : scores) {
if (student.score > maxScore)
maxScore = student.score;
}
return maxScore;
}
int findMin(const std::vector<Student>& scores) {
if (scores.empty()) return INT_MAX; // 空数组,返回整数最大值
int minScore = scores[0].score;
for (const auto& student : scores) {
if (student.score < minScore)
minScore = student.score;
}
return minScore;
}
// 主函数,接收学生成绩数组,然后调用上面的函数
void processScores(std::vector<Student>& scores) {
int maxScore = findMax(scores);
int minScore = findMin(scores);
std::cout << "最大值: " << maxScore << "\n";
std::cout << "最小值: " << minScore << "\n";
// 对数组进行排序,这里使用C++标准库提供的sort函数
std::sort(scores.begin(), scores.end()); // 升序排列
std::cout << "排序后的成绩: \n";
for (const auto& student : scores) {
std::cout << student.score << " ";
}
std::cout << "\n";
}
int main() {
std::vector<Student> studentScores = {85, 92, 76, 98, 88};
processScores(studentScores);
return 0;
}
```
在这个程序中,`processScores`函数接收一个`Student`类型的数组,并通过`findMax`和`findMin`函数找到最大值和最小值,然后对数组进行排序。请注意,这个例子假设了所有学生的分数都是整数。
阅读全文
相关推荐
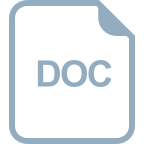
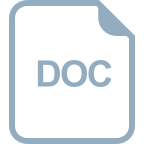
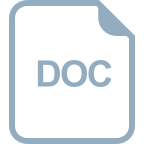
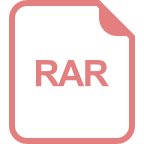
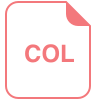




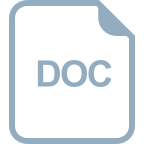








