分别写出socket的服务器端和客户端应用程序代码
时间: 2024-12-24 20:28:34 浏览: 5
在Python中,我们可以使用`socket`模块来创建简单的服务器和客户端程序。这里提供了一个基本的例子:
### 服务器端 (Server.py)
```python
import socket
# 创建套接字
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# 设置主机和端口
host = 'localhost'
port = 12345
# 绑定地址和端口
server_socket.bind((host, port))
# 设置最大连接数
server_socket.listen(5)
print(f"Server is running on {host}:{port}")
while True:
# 等待客户端连接
client_connection, client_address = server_socket.accept()
print(f"Accepted connection from {client_address[0]}:{client_address[1]}")
# 接收客户端数据并回应
request_data = client_connection.recv(1024)
response = "Hello from server!"
# 发送响应给客户端
client_connection.sendall(response.encode('utf-8'))
# 关闭连接
client_connection.close()
```
### 客户端 (Client.py)
```python
import socket
def connect_to_server(host, port):
# 创建套接字
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
try:
# 连接到服务器
client_socket.connect((host, port))
# 发送请求到服务器
message = "Hello, Server!"
client_socket.send(message.encode('utf-8'))
# 接收服务器响应
received_data = client_socket.recv(1024)
print(f"Received: {received_data.decode('utf-8')}")
except Exception as e:
print(f"Error: {e}")
finally:
# 关闭连接
client_socket.close()
if __name__ == "__main__":
host = 'localhost'
port = 12345
connect_to_server(host, port)
```
在这个例子中,服务器等待客户端连接,并发送固定的响应;而客户端向服务器发送消息并接收回复。
阅读全文
相关推荐
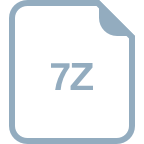
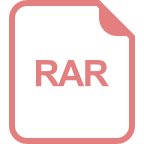
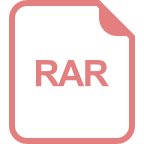

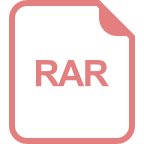
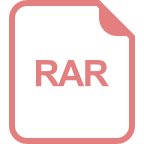
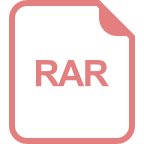
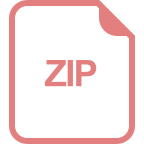
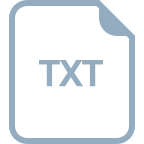
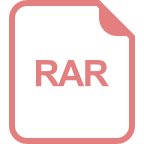
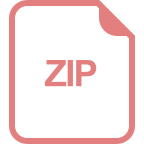
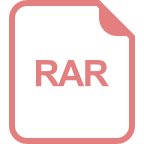
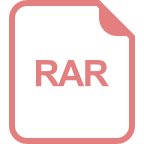
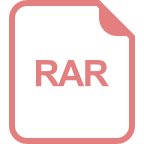
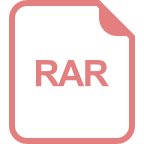
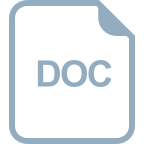
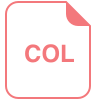

