双向链表的有序插入java
时间: 2024-03-21 17:36:31 浏览: 31
双向链表是一种常见的数据结构,它的每个节点都包含两个指针,一个指向前一个节点,一个指向后一个节点。有序插入是指将元素按照一定的顺序插入到链表中。
在Java中,可以通过自定义一个双向链表类来实现有序插入。以下是一个简单的示例代码:
```java
public class DoublyLinkedList {
private Node head;
private class Node {
int data;
Node prev;
Node next;
public Node(int data) {
this.data = data;
}
}
public void insert(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else if (data < head.data) {
newNode.next = head;
head.prev = newNode;
head = newNode;
} else {
Node current = head;
while (current.next != null && current.next.data < data) {
current = current.next;
}
newNode.next = current.next;
if (current.next != null) {
current.next.prev = newNode;
}
current.next = newNode;
newNode.prev = current;
}
}
public void display() {
Node current = head;
while (current != null) {
System.out.print(current.data + " ");
current = current.next;
}
System.out.println();
}
}
```
使用上述代码,可以创建一个双向链表对象,并通过调用insert方法将元素有序地插入到链表中。最后,调用display方法可以打印出链表的内容。
相关推荐
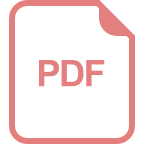
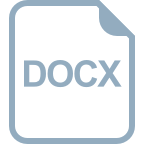
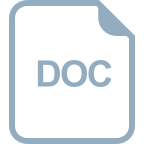














