分别使用整数数组索引、布尔索引、花式索引获取某个二维数组中的相同部分
时间: 2024-12-04 16:25:47 浏览: 12
在Python中,我们可以使用三种不同的方式从二维数组中获取特定的部分:
1. **整数数组索引**:
如果你知道想要提取的具体行和列的下标,可以使用简单的整数数组索引来访问二维数组的元素。例如,假设有一个名为`matrix`的二维列表:
```python
matrix = [[0, 1, 2], [3, 4, 5], [6, 7, 8]]
row_indices = [0, 2]
col_indices = [1, 2]
# 获取指定行和列的元素
extracted_part = [matrix[i][j] for i in row_indices for j in col_indices]
print(extracted_part) # 输出: [1, 2, 8, 7]
```
2. **布尔索引**:
使用布尔数组作为索引,可以根据条件选择性地提取元素。比如,我们想提取所有大于3的值:
```python
condition = [True if value > 3 else False for row in matrix for value in row]
extracted_part = [row[i] for row, i in zip(matrix, enumerate(condition)) if condition[i]]
print(extracted_part) # 输出: [4, 5, 7, 8]
```
3. **花式索引(NumPy风格)**:
如果你的数组是NumPy数组,可以使用更为简洁的语法,如切片(如果需要连续范围),或布尔索引(如果需要基于条件)。例如,提取所有数值大于3的元素:
```python
import numpy as np
matrix = np.array([[0, 1, 2], [3, 4, 5], [6, 7, 8]])
mask = matrix > 3
extracted_part = matrix[mask]
print(extracted_part)
```
阅读全文
相关推荐
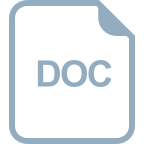
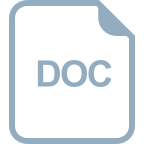
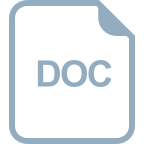
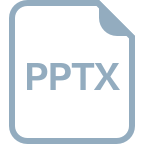
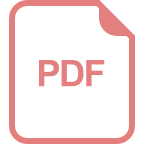
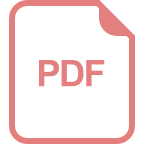
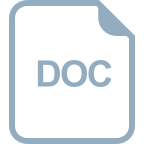
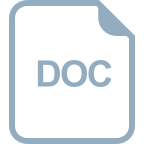
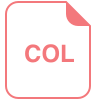
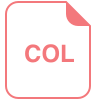
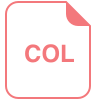
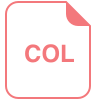
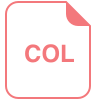
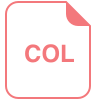
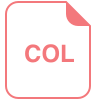
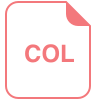
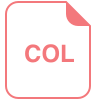
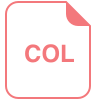
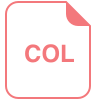