首页写 一段程序要求输入一位学生的生日(年:y1
月:m1, 日:d1),并输入前的日期(年:y1,A:m1,
日:d1),输出该生的实际年龄(周岁
写 一段程序要求输入一位学生的生日(年:y1 月:m1, 日:d1),并输入前的日期(年:y1,A:m1, 日:d1),输出该生的实际年龄(周岁
时间: 2024-11-20 22:41:54 浏览: 5
好的,这里是一个简单的Python程序示例,它会帮助您计算学生实际的年龄:
```python
from datetime import datetime
def calculate_age():
# 获取当前日期
now = datetime.now()
# 输入学生的出生信息
birth_year = int(input("请输入学生的出生年份(y1): "))
birth_month = int(input("请输入出生月份(m1): "))
birth_day = int(input("请输入出生日期(d1): "))
# 创建出生日期的对象
birth_date = datetime(birth_year, birth_month, birth_day)
# 计算年龄
age = now.year - birth_year
if now.month < birth_month or (now.month == birth_month and now.day < birth_day):
age -= 1
print(f"该学生的实际年龄为: {age} 周岁")
calculate_age()
```
在这个程序中,用户会被提示输入学生的出生年、月、日,然后程序会根据当前日期计算出学生的年龄。如果当前还未到他们生日,会减去一岁。
阅读全文
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
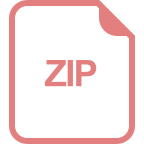
【VRP】遗传算法求解出租车网约车接送客车辆路径规划问题【含Matlab仿真 2153期】.zip
CSDN Matlab武动乾坤上传的资料均有对应的仿真结果图,仿真结果图均是完整代码运行得出,完整代码亲测可用,适合小白;
1、完整的代码压缩包内容
主函数:main.m;
调用函数:其他m文件;无需运行
运行结果效果图;
2、代码运行版本
Matlab 2019b;若运行有误,根据提示修改;若不会,私信博主;
3、运行操作步骤
步骤一:将所有文件放到Matlab的当前文件夹中;
步骤二:双击打开main.m文件;
步骤三:点击运行,等程序运行完得到结果;
4、仿真咨询
如需其他服务,可私信博主或扫描博客文章底部QQ名片;
4.1 博客或资源的完整代码提供
4.2 期刊或参考文献复现
4.3 Matlab程序定制
4.4 科研合作
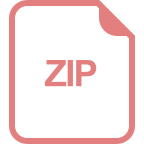
医用废料检测识别针头针管血渍手术刀等 yolov5标记
医用废料检测识别针头针管血渍手术刀等 yolov5标记
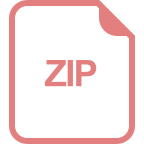
java调用comfyui接口
java调用comfyui接口,通过api json调用,同时可以通过参数替换 api的json中的参数,返回数据包含 :url 、path、base64方式
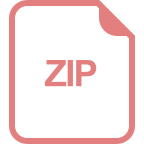
基于Python socket tcp通信,使用tkinter做客户端界面 资料齐全+详细文档.zip
【资源说明】
基于Python socket tcp通信,使用tkinter做客户端界面 资料齐全+详细文档.zip
【备注】
1、该项目是个人高分项目源码,已获导师指导认可通过,答辩评审分达到95分
2、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用!
3、本项目适合计算机相关专业(人工智能、通信工程、自动化、电子信息、物联网等)的在校学生、老师或者企业员工下载使用,也可作为毕业设计、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。
4、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。
欢迎下载,沟通交流,互相学习,共同进步!
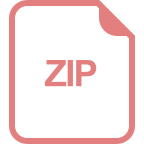
【BAS三维路径规划】蚁群算法结合天牛须算法农用无人机三维路径规划【含Matlab仿真 2341期】.zip
CSDN Matlab武动乾坤上传的资料均有对应的仿真结果图,仿真结果图均是完整代码运行得出,完整代码亲测可用,适合小白;
1、完整的代码压缩包内容
主函数:main.m;
调用函数:其他m文件;无需运行
运行结果效果图;
2、代码运行版本
Matlab 2019b;若运行有误,根据提示修改;若不会,私信博主;
3、运行操作步骤
步骤一:将所有文件放到Matlab的当前文件夹中;
步骤二:双击打开main.m文件;
步骤三:点击运行,等程序运行完得到结果;
4、仿真咨询
如需其他服务,可私信博主或扫描博客文章底部QQ名片;
4.1 博客或资源的完整代码提供
4.2 期刊或参考文献复现
4.3 Matlab程序定制
4.4 科研合作
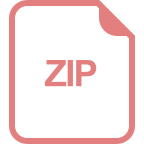
MATLAB新功能:Multi-frame ViewRGB制作彩色图阴影
资源摘要信息:"MULTI_FRAME_VIEWRGB 函数是用于MATLAB开发环境下创建多帧彩色图像阴影的一个实用工具。该函数是MULTI_FRAME_VIEW函数的扩展版本,主要用于处理彩色和灰度图像,并且能够为多种帧创建图形阴影效果。它适用于生成2D图像数据的体视效果,以便于对数据进行更加直观的分析和展示。MULTI_FRAME_VIEWRGB 能够处理的灰度图像会被下采样为8位整数,以确保在处理过程中的高效性。考虑到灰度图像处理的特异性,对于灰度图像建议直接使用MULTI_FRAME_VIEW函数。MULTI_FRAME_VIEWRGB 函数的参数包括文件名、白色边框大小、黑色边框大小以及边框数等,这些参数可以根据用户的需求进行调整,以获得最佳的视觉效果。"
知识点详细说明:
1. MATLAB开发环境:MULTI_FRAME_VIEWRGB 函数是为MATLAB编写的,MATLAB是一种高性能的数值计算环境和第四代编程语言,广泛用于算法开发、数据可视化、数据分析以及数值计算等场合。在进行复杂的图像处理时,MATLAB提供了丰富的库函数和工具箱,能够帮助开发者高效地实现各种图像处理任务。
2. 图形阴影(Shadowing):在图像处理和计算机图形学中,阴影的添加可以使图像或图形更加具有立体感和真实感。特别是在多帧视图中,阴影的使用能够让用户更清晰地区分不同的数据层,帮助理解图像数据中的层次结构。
3. 多帧(Multi-frame):多帧图像处理是指对一系列连续的图像帧进行处理,以实现动态视觉效果或分析图像序列中的动态变化。在诸如视频、连续医学成像或动态模拟等场景中,多帧处理尤为重要。
4. RGB 图像处理:RGB代表红绿蓝三种颜色的光,RGB图像是一种常用的颜色模型,用于显示颜色信息。RGB图像由三个颜色通道组成,每个通道包含不同颜色强度的信息。在MULTI_FRAME_VIEWRGB函数中,可以处理彩色图像,并生成彩色图阴影,增强图像的视觉效果。
5. 参数调整:在MULTI_FRAME_VIEWRGB函数中,用户可以根据需要对参数进行调整,比如白色边框大小(we)、黑色边框大小(be)和边框数(ne)。这些参数影响着生成的图形阴影的外观,允许用户根据具体的应用场景和视觉需求,调整阴影的样式和强度。
6. 下采样(Downsampling):在处理图像时,有时会进行下采样操作,以减少图像的分辨率和数据量。在MULTI_FRAME_VIEWRGB函数中,灰度图像被下采样为8位整数,这主要是为了减少处理的复杂性和加快处理速度,同时保留图像的关键信息。
7. 文件名结构数组:MULTI_FRAME_VIEWRGB 函数使用文件名的结构数组作为输入参数之一。这要求用户提前准备好包含所有图像文件路径的结构数组,以便函数能够逐个处理每个图像文件。
8. MATLAB函数使用:MULTI_FRAME_VIEWRGB函数的使用要求用户具备MATLAB编程基础,能够理解函数的参数和输入输出格式,并能够根据函数提供的用法说明进行实际调用。
9. 压缩包文件名列表:在提供的资源信息中,有两个压缩包文件名称列表,分别是"multi_frame_viewRGB.zip"和"multi_fram_viewRGB.zip"。这里可能存在一个打字错误:"multi_fram_viewRGB.zip" 应该是 "multi_frame_viewRGB.zip"。需要正确提取压缩包中的文件,并且解压缩后正确使用文件名结构数组来调用MULTI_FRAME_VIEWRGB函数。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
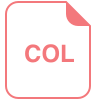
【实战篇:自定义损失函数】:构建独特损失函数解决特定问题,优化模型性能
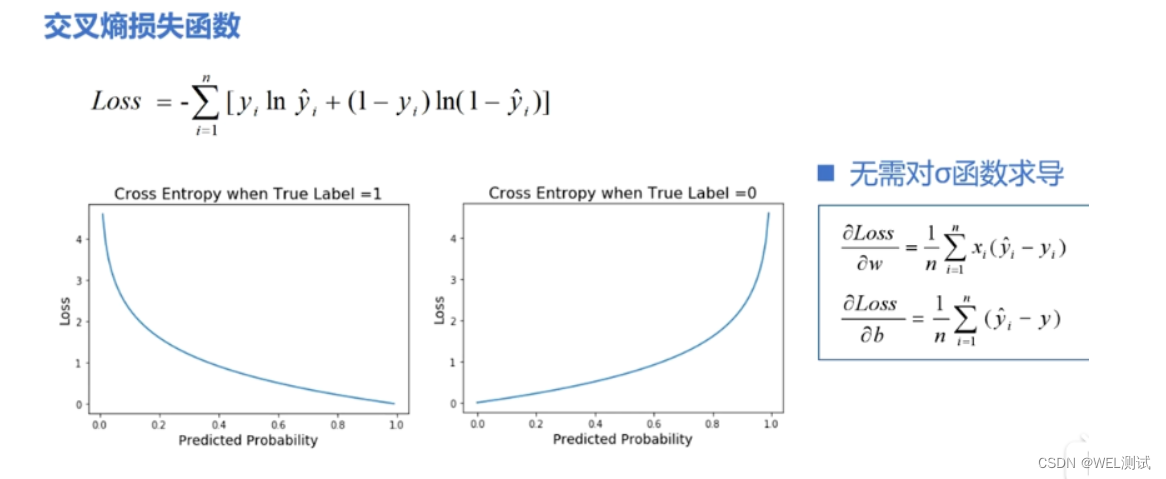
# 1. 损失函数的基本概念与作用
## 1.1 损失函数定义
损失函数是机器学习中的核心概念,用于衡量模型预测值与实际值之间的差异。它是优化算法调整模型参数以最小化的目标函数。
```math
L(y, f(x)) = \sum_{i=1}^{N} L_i(y_i, f(x_i))
```
其中,`L`表示损失函数,`y`为实际值,`f(x)`为模型预测值,`N`为样本数量,`L_i`为第`i`个样本的损失。
## 1.2 损

在Flow-3D中如何根据水利工程的特定需求设定边界条件和进行网格划分,以便准确模拟水流问题?
要在Flow-3D中设定合适的边界条件和进行精确的网格划分,首先需要深入理解水利工程的具体需求和流体动力学的基本原理。推荐参考《Flow-3D水利教程:边界条件设定与网格划分》,这份资料详细介绍了如何设置工作目录,创建模拟文档,以及进行网格划分和边界条件设定的全过程。
参考资源链接:[Flow-3D水利教程:边界条件设定与网格划分](https://wenku.csdn.net/doc/23xiiycuq6?spm=1055.2569.3001.10343)
在设置边界条件时,需要根据实际的水利工程项目来确定,如在模拟渠道流动时,可能需要设定速度边界条件或水位边界条件。对于复杂的
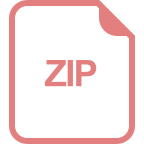
XKCD Substitutions 3-crx插件:创新的网页文字替换工具
资源摘要信息: "XKCD Substitutions 3-crx插件是一个浏览器扩展程序,它允许用户使用XKCD漫画中的内容替换特定网站上的单词和短语。XKCD是美国漫画家兰德尔·门罗创作的一个网络漫画系列,内容通常涉及幽默、科学、数学、语言和流行文化。XKCD Substitutions 3插件的核心功能是提供一个替换字典,基于XKCD漫画中的特定作品(如漫画1288、1625和1679)来替换文本,使访问网站的体验变得风趣并且具有教育意义。用户可以在插件的选项页面上自定义替换列表,以满足个人的喜好和需求。此外,该插件提供了不同的文本替换样式,包括无提示替换、带下划线的替换以及高亮显示替换,旨在通过不同的视觉效果吸引用户对变更内容的注意。用户还可以将特定网站列入黑名单,防止插件在这些网站上运行,从而避免在不希望干扰的网站上出现替换文本。"
知识点:
1. 浏览器扩展程序简介:
浏览器扩展程序是一种附加软件,可以增强或改变浏览器的功能。用户安装扩展程序后,可以在浏览器中添加新的工具或功能,比如自动填充表单、阻止弹窗广告、管理密码等。XKCD Substitutions 3-crx插件即为一种扩展程序,它专门用于替换网页文本内容。
2. XKCD漫画背景:
XKCD是由美国计算机科学家兰德尔·门罗创建的网络漫画系列。门罗以其独特的幽默感著称,漫画内容经常涉及科学、数学、工程学、语言学和流行文化等领域。漫画风格简洁,通常包含幽默和讽刺的元素,吸引了全球大量科技和学术界人士的关注。
3. 插件功能实现:
XKCD Substitutions 3-crx插件通过内置的替换规则集来实现文本替换功能。它通过匹配用户访问的网页中的单词和短语,并将其替换为XKCD漫画中的相应条目。例如,如果漫画1288、1625和1679中包含特定的短语或词汇,这些内容就可以被自动替换为插件所识别并替换的文本。
4. 用户自定义替换列表:
插件允许用户访问选项页面来自定义替换列表,这意味着用户可以根据自己的喜好添加、删除或修改替换规则。这种灵活性使得XKCD Substitutions 3成为一个高度个性化的工具,用户可以根据个人兴趣和阅读习惯来调整插件的行为。
5. 替换样式与用户体验:
插件提供了多种文本替换样式,包括无提示替换、带下划线的替换以及高亮显示替换。每种样式都有其特定的用户体验设计。无提示替换适用于不想分散注意力的用户;带下划线的替换和高亮显示替换则更直观地突出显示了被替换的文本,让更改更为明显,适合那些希望追踪替换效果的用户。
6. 黑名单功能:
为了避免在某些网站上无意中干扰网页的原始内容,XKCD Substitutions 3-crx插件提供了黑名单功能。用户可以将特定的域名加入黑名单,防止插件在这些网站上运行替换功能。这样可以保证用户在需要专注阅读的网站上,如工作相关的平台或个人兴趣网站,不会受到插件内容替换的影响。
7. 扩展程序与网络安全:
浏览器扩展程序可能会涉及到用户数据和隐私安全的问题。因此,安装和使用任何第三方扩展程序时,用户都应该确保来源的安全可靠,避免授予不必要的权限。同时,了解扩展程序的权限范围和它如何处理用户数据对于保护个人隐私是至关重要的。
通过这些知识点,可以看出XKCD Substitutions 3-crx插件不仅仅是一个简单的文本替换工具,而是一个结合了个人化定制、交互体验设计以及用户隐私保护的实用型扩展程序。它通过幽默风趣的XKCD漫画内容为用户带来不一样的网络浏览体验。