试编写一个以三元组形式输出用十字链表表示的稀蔬知下标的算法
时间: 2024-10-24 22:07:51 浏览: 32
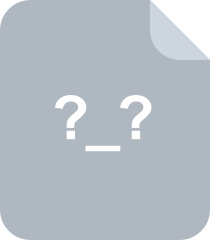
以三元组形式输出用十字链表表示的矩阵的非零元素及其下标
十字链表是一种数据结构,它将稀疏矩阵的非零元素以双向链表的形式存储。在这种结构中,每个节点包含一个值、行索引和列索引,仅存储非零元素,并通过连接相邻的非零元素来减少存储空间。下面是使用Python编写的一个简单算法,用于从稀疏矩阵生成十字链表并输出以三元组的形式:
```python
class Node:
def __init__(self, value, row, col):
self.value = value
self.row = row
self.col = col
self.next_row = None
self.prev_row = None
self.next_col = None
self.prev_col = None
def create_crooked_link_list(matrix):
if not matrix or not any(matrix):
return None
# 初始化头结点
first_node = Node(matrix[0][0], 0, 0)
# 创建链表节点
current = first_node
for i in range(len(matrix)):
for j in range(len(matrix[i])):
if matrix[i][j] != 0:
new_node = Node(matrix[i][j], i, j)
if i > 0 and matrix[i - 1][j] != 0: # 如果上一行有元素,则链接
current.prev_row = new_node
new_node.next_row = current
if j > 0 and matrix[i][j - 1] != 0: # 如果左列有元素,则链接
current.prev_col = new_node
new_node.next_col = current
# 更新当前节点
current = new_node
return first_node
def output_triples(node):
while node is not None:
print((node.row, node.col, node.value))
node = node.next_col if node.col < len(node.matrix) - 1 else node.next_row if node.row < len(node.matrix) - 1 else None
# 示例稀疏矩阵
sparse_matrix = [[0, 1, 0], [0, 0, 2], [0, 0, 0]]
link_list_head = create_crooked_link_list(sparse_matrix)
output_triples(link_list_head)
```
在这个例子中,`create_crooked_link_list`函数创建了十字链表,而`output_triples`函数遍历链表并输出以三元组形式的元素。请注意,这个示例假设输入的矩阵是一个二维列表,其中非零元素用整数表示。
阅读全文
相关推荐
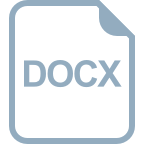
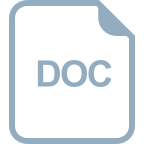

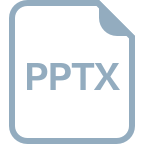
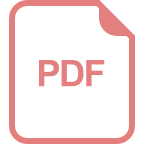
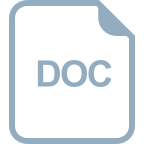
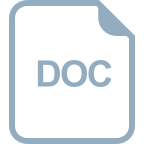
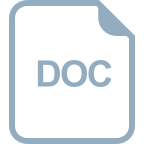
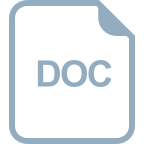



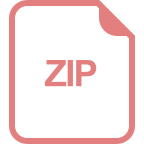
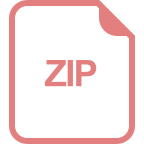
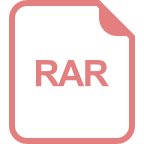
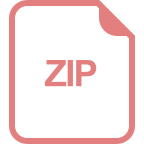