基于STM32F103C8T6使用库函数驱动DAC8563能够输出有效值为1.00V的正弦波,写出它的代码,分别存为main.c,sin.c和sin.h
时间: 2024-04-15 17:30:36 浏览: 212
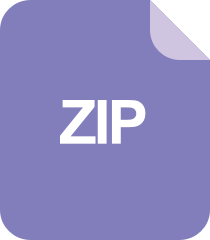
STM32F103用DAC功能输出正弦波

好的,下面是代码示例:
main.c:
```c
#include "stm32f10x.h"
#include "sin.h"
int main(void)
{
// 初始化DAC
DAC_Init();
while (1)
{
// 输出1.00V的正弦波
DAC_OutputSinWave(1.00);
}
}
```
sin.h:
```c
#ifndef __SIN_H
#define __SIN_H
// DAC初始化函数
void DAC_Init(void);
// 输出指定电压的正弦波
void DAC_OutputSinWave(float voltage);
#endif /* __SIN_H */
```
sin.c:
```c
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_dac.h"
#include "sin.h"
// 正弦波数据表,假设表长度为100
const uint16_t sinTable[100] = { .... }; // 此处为正弦波数据表,可以自行生成或者使用已有数据表
void DAC_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
DAC_InitTypeDef DAC_InitStructure;
// 使能GPIO和DAC时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_DAC, ENABLE);
// 配置DAC引脚为模拟输出
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AIN;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 初始化DAC
DAC_InitStructure.DAC_Trigger = DAC_Trigger_None;
DAC_InitStructure.DAC_WaveGeneration = DAC_WaveGeneration_None;
DAC_InitStructure.DAC_LFSRUnmask_TriangleAmplitude = DAC_LFSRUnmask_Bit0;
DAC_InitStructure.DAC_OutputBuffer = DAC_OutputBuffer_Disable;
DAC_Init(DAC_Channel_1, &DAC_InitStructure);
// 使能DAC通道1
DAC_Cmd(DAC_Channel_1, ENABLE);
// 启动DAC
DAC_DMACmd(DAC_Channel_1, ENABLE);
DAC_SoftwareTriggerCmd(DAC_Channel_1, ENABLE);
}
void DAC_OutputSinWave(float voltage)
{
uint16_t i;
uint16_t dacValue;
// 计算DAC输出值
dacValue = (uint16_t)((voltage / 3.3) * 4095);
// 输出正弦波
for (i = 0; i < 100; i++)
{
DAC_SetChannel1Data(DAC_Align_12b_R, sinTable[i] * dacValue / 100);
delay_ms(10); // 延时10ms,控制正弦波频率
}
}
```
注意:上述代码仅为示例,具体的正弦波数据表以及延时函数需要根据实际需求进行修改或添加。
阅读全文
相关推荐
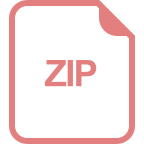
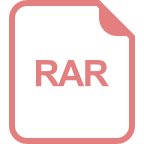
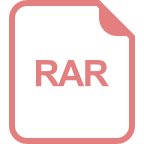
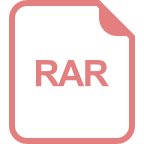
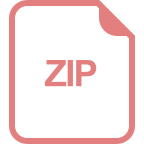
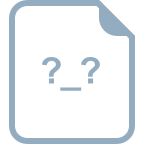
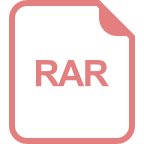
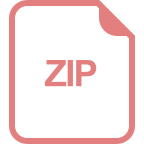
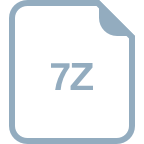
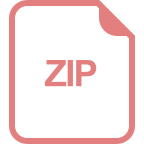
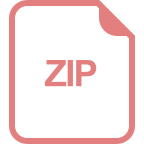
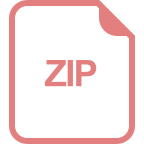
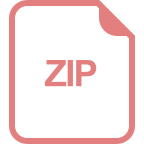
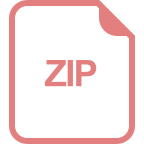
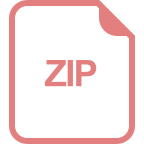