linux中c语言使用文件IO,向文件中第 10 位置处写一个字符,在文件此时的位置,后 20个位置处,写一行字符串hello进去,求此时文件的长度。
时间: 2024-12-08 08:21:44 浏览: 9
在Linux中使用C语言进行文件I/O操作时,你可以使用标准库函数如`fseek`、`fwrite`和`ftell`等来实现你所描述的需求。下面是一个简化的步骤说明:
1. 打开文件:首先使用`fopen`打开你要操作的文件,如果文件不存在则需要创建。
```c
FILE *file = fopen("example.txt", "r+"); // "r+"模式可以读写
if (file == NULL) {
perror("Error opening file");
exit(EXIT_FAILURE);
}
```
2. 移动到指定位置:使用`fseek`将文件指针移动到第10个字节的位置。
```c
off_t position = 9; // 字节偏移从0开始计数,所以是9不是10
if (fseek(file, position, SEEK_SET) != 0) {
perror("Error seeking in file");
fclose(file);
exit(EXIT_FAILURE);
}
```
3. 写入字符:接下来,使用`putc`或`fputc`写入一个字符。
```c
char char_to_write = 'H'; // 或者你想写的任意字符
if (fputc(char_to_write, file) == EOF) {
perror("Error writing to file");
fclose(file);
exit(EXIT_FAILURE);
}
```
4. 写入字符串:跳过已写入字符的位置,然后继续移动到20个字节后并写入"hello"。
```c
position += sizeof(char); // 跳过刚写的字符
if (fseek(file, position, SEEK_SET) != 0) {
perror("Error seeking in file after write");
fclose(file);
exit(EXIT_FAILURE);
}
// 注意这里的字符串需要加上结束符'\0'
const char *str = "hello\0";
size_t str_len = strlen(str);
if (fwrite(str, 1, str_len, file) != str_len) {
perror("Error writing string to file");
fclose(file);
exit(EXIT_FAILURE);
}
```
5. 求文件长度:最后,使用`ftell`获取当前文件指针位置作为新长度,再关闭文件。
```c
off_t new_file_length;
new_file_length = ftell(file);
fclose(file);
printf("New file length: %lld bytes\n", new_file_length);
```
如果你想要立即得到文件的确切长度而不是计算,可以在创建文件后直接使用`lseek`设置到末尾,然后再调用`fseek`和`fread`完成上述操作。注意这会覆盖原有内容。
阅读全文
相关推荐
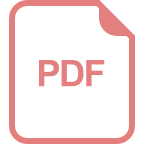
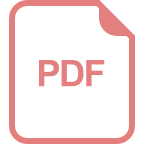
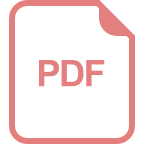

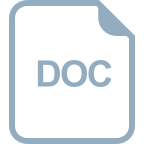
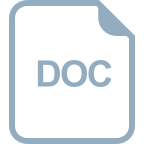
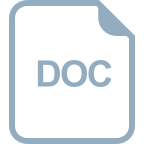
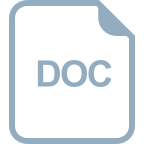
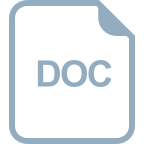




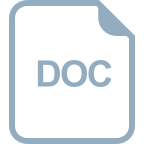
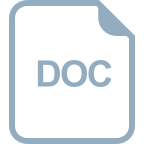
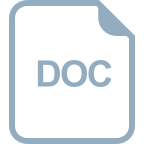
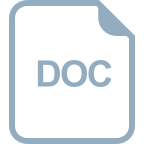

