请用C语言编程实现以下功能: 删除单向链表中的重复节点,并打印结果
时间: 2024-09-23 19:16:18 浏览: 47
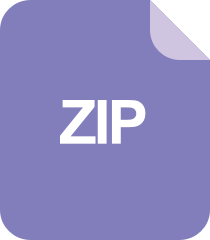
c语言编程题之链表操作删除链表中的节点.zip
在C语言中,我们可以使用结构体表示链表节点,同时遍历链表并删除重复的元素。以下是简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
// 创建新节点
ListNode* createNode(int val) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = val;
newNode->next = NULL;
return newNode;
}
// 比较两个节点是否相等
int areEqual(const ListNode* a, const ListNode* b) {
return a && b && a->data == b->data;
}
// 删除重复节点
ListNode* deleteDuplicates(ListNode* head) {
if (!head || !head->next) {
return head;
}
ListNode* current = head;
while (current->next) {
if (areEqual(current, current->next)) {
// 如果当前节点与下一个节点相等,跳过这个节点
current->next = current->next->next;
} else {
current = current->next; // 否则继续向前移动
}
}
return head;
}
// 打印链表
void printList(ListNode* node) {
while (node) {
printf("%d -> ", node->data);
node = node->next;
}
printf("NULL\n");
}
int main() {
// 创建示例链表
ListNode* list = createNode(1);
list->next = createNode(2);
list->next->next = createNode(3);
list->next->next->next = createNode(4);
list->next->next->next->next = createNode(2); // 包含重复节点
printf("Original List:\n");
printList(list);
// 删除重复节点
list = deleteDuplicates(list);
printf("\nList after removing duplicates:\n");
printList(list);
return 0;
}
```
在这个例子中,我们首先创建了一个包含重复值的链表,然后使用`deleteDuplicates`函数删除了重复节点,最后打印出处理后的链表。
阅读全文
相关推荐
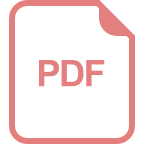
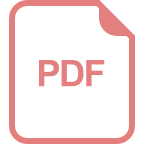

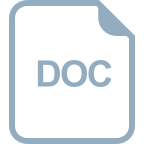
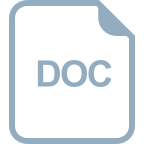

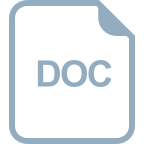
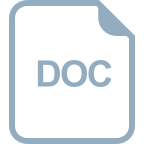
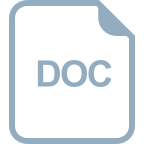
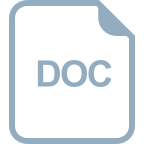
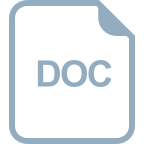
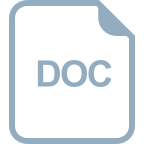
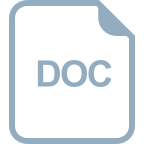
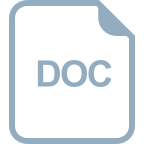
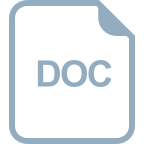


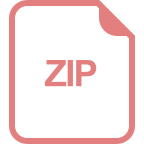