用c++语言编写完整程序实现链表的初始化、查找、插入、删除、遍历等操作
时间: 2024-12-06 19:23:35 浏览: 45
在C++中,我们可以使用结构体表示链表节点,以下是一个简化的示例,展示了如何实现链表的初始化、查找、插入、删除和遍历:
```cpp
#include <iostream>
// 链表节点结构
struct ListNode {
int val; // 节点值
ListNode* next; // 指向下一个节点的指针
ListNode(int x) : val(x), next(nullptr) {} // 构造函数
};
// 初始化一个长度为n的链表
ListNode* createList(int n) {
ListNode* head = new ListNode(0); // 创建头节点
for (int i = 1; i < n; ++i) {
ListNode* newNode = new ListNode(i);
head->next = newNode;
head = newNode;
}
return head;
}
// 查找链表中某个值
bool search(ListNode* head, int target) {
ListNode* current = head;
while (current != nullptr) {
if (current->val == target)
return true;
current = current->next;
}
return false;
}
// 插入元素到指定位置
void insert(ListNode*& head, int x, int index) {
if (index == 0) {
ListNode* newNode = new ListNode(x);
newNode->next = head;
head = newNode;
} else {
ListNode* prev = nullptr;
ListNode* current = head;
for (int i = 0; i < index - 1 && current != nullptr; ++i) {
prev = current;
current = current->next;
}
if (prev != nullptr)
prev->next = new ListNode(x);
else
head = new ListNode(x);
current->next = new ListNode(0);
current->next->next = head;
}
}
// 删除指定位置的元素
void deleteElement(ListNode*& head, int index) {
if (index == 0) {
ListNode* temp = head;
head = head->next;
delete temp;
} else {
ListNode* prev = nullptr;
ListNode* current = head;
for (int i = 0; i < index - 1 && current != nullptr; ++i) {
prev = current;
current = current->next;
}
if (prev == nullptr)
return; // 如果越界,不做操作
prev->next = current->next;
delete current;
}
}
// 遍历链表并打印值
void printList(ListNode* head) {
ListNode* current = head;
while (current != nullptr) {
std::cout << current->val << " -> ";
current = current->next;
}
std::cout << "NULL" << std::endl;
}
int main() {
// 示例操作
int n = 5;
ListNode* list = createList(n);
int target = 3;
bool found = search(list, target);
std::cout << "Find " << target << ": " << (found ? "Yes" : "No") << std::endl;
int x = 10, index = 2;
insert(list, x, index);
printList(list);
int delIndex = 2;
deleteElement(list, delIndex);
printList(list);
return 0;
}
```
这个例子展示了如何创建、操作和遍历一个简单的整数链表。记得根据实际需求调整代码细节。运行`main()`函数可以看到各个操作的效果。
阅读全文
相关推荐













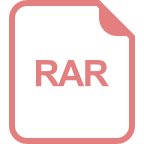




