编写完整程序LinkList.c或LinkList.cpp实现链表的初始化、查找、插入、删除、遍历等操作。具体实现要求:
时间: 2024-11-20 10:42:35 浏览: 25
在C或C++中,我们通常会使用结构体(struct)和函数来实现链表的基本操作。这里我将提供一个简单的单链表实现的例子,包括初始化、查找、插入、删除和遍历功能。假设我们有一个`Node`结构体表示链表节点,以及一个`LinkedList`类处理整个链表。
**在C语言中:**
```c
#include <stdio.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表为空
void initLinkedList(Node** head) {
*head = NULL;
}
// 查找节点
Node* search(Node* head, int key) {
Node* curr = head;
while (curr != NULL && curr->data != key) {
curr = curr->next;
}
return curr;
}
// 插入节点
void insertNode(Node** head, int key) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = key;
newNode->next = *head;
*head = newNode;
}
// 删除节点
void deleteNode(Node** head, int key) {
Node* temp = *head;
Node* prev = NULL;
if (temp != NULL && temp->data == key) {
*head = temp->next; // 如果头节点就是目标,直接替换
free(temp);
return;
}
while (temp != NULL && temp->data != key) {
prev = temp;
temp = temp->next;
}
if (temp == NULL) return; // 没找到目标
prev->next = temp->next;
free(temp);
}
// 遍历链表并打印节点值
void printList(Node* head) {
Node* curr = head;
while (curr != NULL) {
printf("%d -> ", curr->data);
curr = curr->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL;
initLinkedList(&head);
// 插入、查找、删除和遍历操作...
insertNode(&head, 5); // 添加节点5
printList(head); // 打印链表
Node* found = search(head, 5); // 查找节点5
if (found) {
deleteNode(&head, 5); // 删除节点5
} else {
printf("Node not found.\n");
}
return 0;
}
```
**在C++中:**
```cpp
#include <iostream>
class Node {
public:
int data;
Node* next;
};
// 类实现
class LinkedList {
private:
Node* head;
public:
LinkedList() : head(nullptr) {}
void insert(int key) {
Node* newNode = new Node{key, head};
head = newNode;
}
Node* search(int key) {
Node* curr = head;
while (curr && curr->data != key) {
curr = curr->next;
}
return curr;
}
void remove(int key) {
if (!head || head->data == key) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* prev = nullptr;
for (Node* curr = head; curr && curr->data != key; prev = curr, curr = curr->next) {}
if (curr == nullptr) return; // 没有找到
if (prev) prev->next = curr->next;
delete curr;
}
void traverse() {
Node* curr = head;
while (curr) {
std::cout << curr->data << " -> ";
curr = curr->next;
}
std::cout << "NULL\n";
};
};
int main() {
LinkedList list;
list.insert(5); // 插入节点5
list.traverse(); // 打印链表
Node* found = list.search(5); // 查找节点5
if (found) {
list.remove(5); // 删除节点5
} else {
std::cout << "Node not found.\n";
}
return 0;
}
```
阅读全文
相关推荐
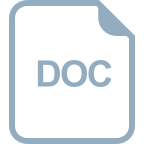
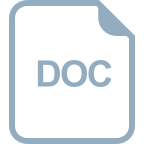
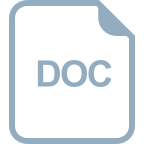



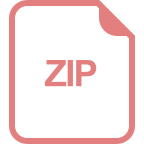
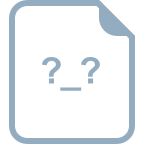
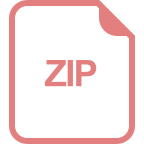
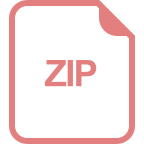
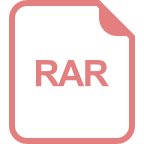





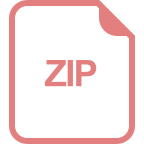

