使用【C++】写一个复杂的【成语接龙游戏】的代码
时间: 2024-12-20 13:19:12 浏览: 9
编写一个复杂的成语接龙游戏涉及到多个步骤,包括但不限于定义数据结构存储成语、处理用户输入、检查接龙规则等。以下是一个简单的示例,使用C++来实现基本功能:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <unordered_map>
// 定义成语的数据结构
struct Acronym {
std::string acronym;
std::string meaning;
};
class Game {
private:
// 存储成语字典,这里简单用map表示,实际应用可能需要更复杂的数据结构
std::unordered_map<std::string, Acronym> dictionary;
// 成语接龙队列
std::vector<std::string> chain;
public:
void initDictionary() { ... } // 加载成语字典
bool isLegal(const std::string& next) const { ... } // 检查下一个成语是否合法
void startGame() {
// 初始化游戏开始
if (!initDictionary()) {
std::cerr << "Error loading dictionary." << std::endl;
return;
}
std::cout << "Welcome to the Chinese idiom game! Type an idiom starting with the last word of the previous one.\n";
std::string input;
chain.push_back(dictionary.begin()->first); // 开始链
while (true) {
std::cin >> input;
if (isLegal(input)) {
chain.push_back(input);
std::cout << "Correct! Your chain so far: " << chain.back() << "\n";
} else {
std::cout << "Invalid idiom. Try again.\n";
}
}
}
};
int main() {
Game game;
game.startGame();
return 0;
}
```
**注意**:
- `initDictionary()` 函数用于加载成语字典,这通常需要外部文件或网络接口,此处省略了具体实现。
- `isLegal(const std::string& next)` 函数需根据字典验证输入的成语是否符合接龙规则,比如首字母必须匹配上一个成语的尾字母。
在这个例子中,我们假设每个成语只有一个正确的接龙形式。在实际游戏中,可能会有多个合法接龙选项(比如同音字),并需要处理这种情况。
**相关问题--:**
1. 如何设计一个高效查找成语接龙下一位的算法?
2. 怎样扩展这个程序,使其支持同音字接龙?
3. 游戏如何保存和加载进度?
4. 如何增加难度级别,例如限制输入次数或时间?
阅读全文
相关推荐
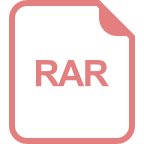
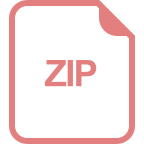
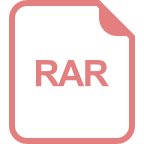












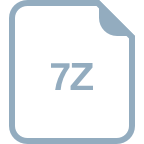
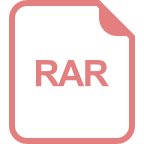