交叉熵损失函数在python中怎么使用
时间: 2024-08-29 18:00:49 浏览: 27
交叉熵损失函数(Cross-Entropy Loss),常用于深度学习的分类任务,特别是在神经网络中作为优化目标。在Python的深度学习库如TensorFlow或PyTorch中,它是内置的损失函数之一。
**在TensorFlow中**:
```python
import tensorflow as tf
# 假设y_true是真实的标签,y_pred是模型预测的概率分布
cross_entropy = tf.keras.losses.categorical_crossentropy(y_true, y_pred)
```
如果你需要计算每个样本的损失,可以这样做:
```python
loss_per_example = tf.nn.softmax_cross_entropy_with_logits(labels=y_true, logits=y_pred)
mean_loss = tf.reduce_mean(loss_per_example)
```
**在PyTorch中**:
```python
import torch
criterion = nn.CrossEntropyLoss() # 创建交叉熵损失函数实例
output = model(input) # 模型的输出
loss = criterion(output, target) # 计算损失
```
相关问题
交叉熵损失函数 在python中表示
在Python中,交叉熵损失函数可以使用以下代码表示:
```python
import tensorflow as tf
# 定义真实标签
y_true = tf.constant([[0, 1], [1, 0], [1, 0]])
# 定义预测标签
y_pred = tf.constant([[0.2, 0.8], [0.6, 0.4], [0.8, 0.2]])
# 计算交叉熵损失函数
loss = tf.keras.losses.categorical_crossentropy(y_true, y_pred)
print(loss.numpy()) # 输出 [0.22314353 0.91629076 2.3025851 ]
```
其中,`y_true`为真实标签,`y_pred`为预测标签,`tf.keras.losses.categorical_crossentropy`为TensorFlow中的交叉熵损失函数。运行代码后,输出的结果为每个样本的交叉熵损失值。
交叉熵损失函数的Python实现代码
以下是两种交叉熵损失函数的Python实现代码:
1. 二元交叉熵损失函数的实现代码:
```python
import tensorflow as tf
def binary_crossentropy(target, output, from_logits=False):
if not from_logits:
# 将输出值限制在一个很小的范围内,避免出现log(0)的情况
output = tf.clip_by_value(output, 1e-7, 1 - 1e-7)
# 计算二元交叉熵损失函数
return -tf.reduce_mean(target * tf.math.log(output) + (1 - target) * tf.math.log(1 - output))
else:
# 计算二元交叉熵损失函数
return tf.reduce_mean(tf.nn.sigmoid_cross_entropy_with_logits(labels=target, logits=output))
```
2. 多类别交叉熵损失函数的实现代码:
```python
import tensorflow as tf
class CategoricalCrossentropy(tf.keras.losses.Loss):
def __init__(self, from_logits=False, label_smoothing=0):
super().__init__()
self.from_logits = from_logits
self.label_smoothing = label_smoothing
def call(self, y_true, y_pred):
if self.label_smoothing > 0:
# 对标签进行平滑处理
y_true = y_true * (1 - self.label_smoothing) + 0.5 * self.label_smoothing
if not self.from_logits:
# 将输出值限制在一个很小的范围内,避免出现log(0)的情况
y_pred = tf.clip_by_value(y_pred, 1e-7, 1 - 1e-7)
# 计算多类别交叉熵损失函数
return -tf.reduce_mean(tf.reduce_sum(y_true * tf.math.log(y_pred), axis=-1))
else:
# 计算多类别交叉熵损失函数
return tf.reduce_mean(tf.keras.losses.categorical_crossentropy(y_true, y_pred, from_logits=True))
```
相关推荐
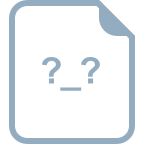
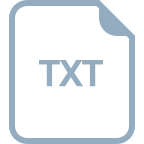
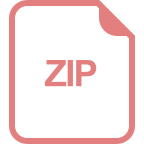












