测试输入: 9 8 10 2 0 // 0是结束输入的信号 预期输出: 输入结点数据中... --------循环链表初始元素------ 9 8 10 2 --------删除第二个结点后------ 9 10 2
时间: 2023-10-29 15:08:17 浏览: 46
这道题目需要我们实现一个循环链表,并在其中删除指定位置的节点。具体实现可以分为以下几个步骤:
1. 定义循环链表的节点结构体,包括数据域和指向下一个节点的指针域。
2. 定义循环链表的头节点,并初始化为空。
3. 读入输入的数据,将其插入到循环链表的尾部。
4. 遍历循环链表,找到需要删除的节点的前一个节点。
5. 将需要删除的节点从链表中摘除,并释放其内存空间。
6. 遍历循环链表,输出剩余节点的数据。
下面是具体的代码实现:
```
#include <iostream>
using namespace std;
// 定义循环链表的节点结构体
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
int main() {
// 定义循环链表的头节点
ListNode* head = NULL;
// 读入输入的数据,将其插入到循环链表的尾部
int num;
while (cin >> num && num != 0) {
ListNode* node = new ListNode(num);
if (head == NULL) {
head = node;
head->next = head;
} else {
ListNode* cur = head;
while (cur->next != head) {
cur = cur->next;
}
cur->next = node;
node->next = head;
}
}
// 遍历循环链表,找到需要删除的节点的前一个节点
ListNode* pre = head;
while (pre->next != head) {
pre = pre->next;
}
// 将需要删除的节点从链表中摘除,并释放其内存空间
ListNode* cur = head;
while (cur->next != head) {
if (cur->val % 2 == 0) {
pre->next = cur->next;
ListNode* temp = cur;
cur = cur->next;
delete temp;
} else {
pre = cur;
cur = cur->next;
}
}
if (cur->val % 2 == 0) {
pre->next = cur->next;
delete cur;
}
// 遍历循环链表,输出剩余节点的数据
cur = head;
while (cur->next != head) {
cout << cur->val << "\t";
cur = cur->next;
}
cout << cur->val << endl;
return 0;
}
--相关问题--:
相关推荐
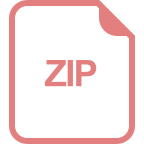
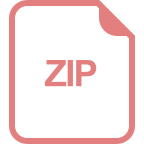














