stm32串口双向通信完整代码
时间: 2024-01-05 11:21:08 浏览: 43
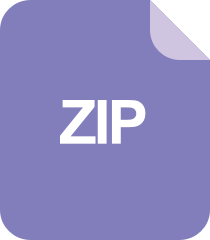
stm32 最完整的串口通讯代码

以下是一个示例的STM32串口双向通信的完整代码:
```c
#include "stm32f10x.h"
void USART1_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
// 使能USART1和GPIOA的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1的引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; // USART1_TX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; // USART1_RX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置USART1的参数
USART_InitStructure.USART_BaudRate = 9600;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char ch)
{
// 等待发送缓冲区为空
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
// 发送一个字符
USART_SendData(USART1, (uint16_t)ch);
}
char USART1_ReceiveChar(void)
{
// 等待接收缓冲区非空
while (USART_GetFlagStatus(USART1, USART_FLAG_RXNE) == RESET);
// 读取接收到的字符
return (char)USART_ReceiveData(USART1);
}
int main(void)
{
char receivedChar;
// 初始化USART1
USART1_Init();
while (1)
{
// 接收字符
receivedChar = USART1_ReceiveChar();
// 处理接收到的字符
// ...
// 发送字符
USART1_SendChar(receivedChar);
}
}
```
阅读全文
相关推荐
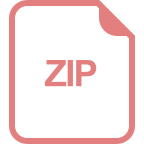
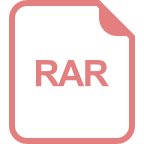
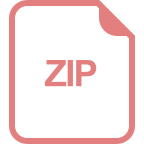
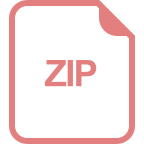
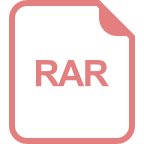
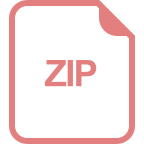
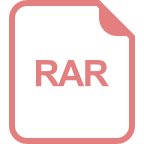
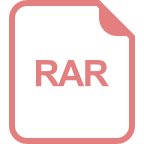
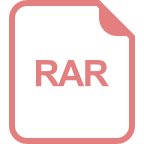
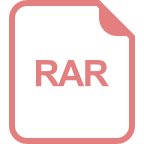
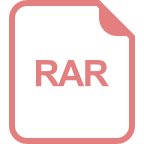
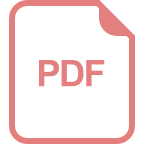
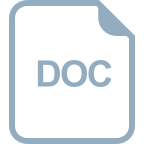




