tcn中的注意力推荐
时间: 2023-09-08 16:03:46 浏览: 56
TCN(Temporal Convolutional Network)是一种用于序列数据建模的深度学习模型。而注意力机制(Attention)是一种可以提升模型性能的技术。
TCN中的注意力推荐就是在TCN模型中引入注意力机制来提高模型的性能。注意力机制允许模型在处理序列数据时对输入的不同部分赋予不同的权重和关注度,以便更好地捕捉重要的信息和模式。
在TCN中引入注意力机制可以有多种方式。一种常见的方法是在TCN的卷积层后添加一个注意力层。这个注意力层可以通过学习权重矩阵,将不同时间步的输入特征进行加权,以便更加关注具有更高重要性的时间步。
另一种方法是引入多头注意力机制,即在TCN模型的多个卷积层后分别添加多个注意力头。每个注意力头可以关注序列数据中的不同方面和特征,从而提取更加丰富和准确的表示。
通过引入注意力机制,TCN模型可以更好地适应序列数据中的长期依赖关系和动态模式,从而改善模型的建模能力和预测性能。注意力机制可以有效地提取序列数据中的重要信息,并且可以根据不同的输入自动调整权重,使得模型更加灵活和准确。
总之,TCN中的注意力推荐是一种通过引入注意力机制来提升TCN模型性能的方法。它可以在处理序列数据时更好地捕捉关键信息和动态模式,从而提高模型的建模能力和预测准确性。
相关问题
基于注意力机制的TcN
基于注意力机制的TCN(Temporal Convolutional Network)是一种用于序列建模的网络结构。它结合了时间卷积网络(TCN)和注意力机制,能够捕捉序列中的相关特征并提取重要信息。
在基于注意力机制的TCN中,有两个关键部分:时间注意力(TA)和增强残差(ER)。时间注意力用于捕捉序列中的相关特征,它通过对序列中的不同时间步进行加权,使得网络能够更加关注重要的时间步。增强残差用于提取浅层的重要信息并迁移到深层,它通过残差连接的方式将浅层的信息传递给深层,从而增强网络的表达能力。
基于注意力机制的TCN在序列建模任务中具有很好的效果。它能够自动学习序列中的相关特征,并且能够提取重要的信息进行预测。这种网络结构在时间序列预测、自然语言处理等领域都有广泛的应用。
参考资料:
Hongyan, L., et al. (2020). Temporal Convolutional Attention-based Network For Sequence Modeling.
目录 时序预测 | Python实现Attention-TCN注意力机制时间卷积神经网络的多元时间序列预测预测效果基本介绍环境配置程序设计模型效果参考资料
tcn加注意力机制进行信用卡欺诈检测代码
以下是使用TCN和注意力机制进行信用卡欺诈检测的代码示例。
首先,我们需要导入所需的库和模块。
```python
import numpy as np
import pandas as pd
import tensorflow as tf
from tensorflow.keras.layers import Input, Dense, Dropout, SpatialDropout1D, Conv1D, MaxPooling1D, Flatten, \
concatenate, Attention
from tensorflow.keras.models import Model
from tensorflow.keras.callbacks import EarlyStopping
from sklearn.metrics import confusion_matrix, classification_report
from sklearn.model_selection import train_test_split
```
然后,我们读取数据集并将其拆分为训练集和测试集。
```python
data = pd.read_csv('creditcard.csv')
X = data.drop(['Class'], axis=1)
y = data['Class']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
```
接下来,我们将数据转换为3D张量以适应TCN模型的输入形状。我们还将标准化输入以提高模型的性能。
```python
def prepare_data(X, y):
n_features = X.shape[1]
X = np.array(X).reshape((-1, n_features, 1))
y = np.array(y)
X_mean = X.mean(axis=0)
X_std = X.std(axis=0)
X = (X - X_mean) / X_std
return X, y
X_train, y_train = prepare_data(X_train, y_train)
X_test, y_test = prepare_data(X_test, y_test)
```
然后,我们定义TCN模型。这个模型由一系列卷积层和空间丢失层组成,最后通过一个全局最大池化层来提取特征。然后,我们使用注意力机制来加权这些特征,并将其馈入一个密集层进行最终分类。
```python
def build_model(n_features, kernel_size=3):
input_layer = Input(shape=(n_features, 1))
conv1 = Conv1D(filters=64, kernel_size=kernel_size, activation='relu')(input_layer)
conv2 = Conv1D(filters=64, kernel_size=kernel_size, activation='relu')(conv1)
dropout1 = SpatialDropout1D(rate=0.2)(conv2)
maxpool1 = MaxPooling1D(pool_size=2)(dropout1)
conv3 = Conv1D(filters=128, kernel_size=kernel_size, activation='relu')(maxpool1)
conv4 = Conv1D(filters=128, kernel_size=kernel_size, activation='relu')(conv3)
dropout2 = SpatialDropout1D(rate=0.2)(conv4)
maxpool2 = MaxPooling1D(pool_size=2)(dropout2)
conv5 = Conv1D(filters=256, kernel_size=kernel_size, activation='relu')(maxpool2)
conv6 = Conv1D(filters=256, kernel_size=kernel_size, activation='relu')(conv5)
dropout3 = SpatialDropout1D(rate=0.2)(conv6)
attention = Attention()([dropout3, dropout3])
flatten = Flatten()(attention)
dense1 = Dense(units=128, activation='relu')(flatten)
dense2 = Dense(units=1, activation='sigmoid')(dense1)
model = Model(inputs=input_layer, outputs=dense2)
return model
model = build_model(n_features=X_train.shape[1])
```
接下来,我们编译模型并训练它。
```python
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
early_stopping = EarlyStopping(monitor='val_loss', patience=3)
model.fit(X_train, y_train, epochs=10, batch_size=64, validation_split=0.2, callbacks=[early_stopping])
```
最后,我们评估模型的性能并输出分类报告和混淆矩阵。
```python
y_pred = model.predict(X_test)
y_pred = np.round(y_pred)
print(classification_report(y_test, y_pred))
print(confusion_matrix(y_test, y_pred))
```
相关推荐
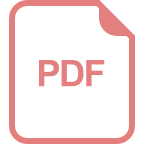
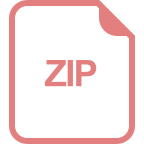












