softmax手写数字识别 曲线图
时间: 2023-11-14 09:04:12 浏览: 33
softmax手写数字识别任务的曲线图无法使用线性方程来刻画,因为输入像素和输出数字标签之间的关系不是线性的。softmax函数用于将输出层的原始分数转化为概率分布,以确定最可能的数字标签。该函数将原始分数转换为概率值,且所有概率值的总和为1。因此,softmax手写数字识别任务的曲线图应该是一个非线性的曲线。
相关问题
用softmax手写数字识别
使用softmax进行手写数字识别是一种常见的机器学习方法。softmax是一种用于多分类问题的激活函数,它将一个向量映射为另一个向量,其中每个元素都表示该类别的概率。在手写数字识别中,我们可以将每个手写数字图像看作一个向量,然后使用softmax函数将其映射为一个概率向量,其中每个元素表示该图像属于某个数字的概率。通过训练模型,我们可以调整模型的参数,使得模型能够准确地预测手写数字图像的类别。
Softmax实现手写数字识别
mnist手写数字识别softmax是一种用于对手写数字图像进行分类的方法,它将每个数字图像分成10类,并将每个类的概率作为输出。该方法基于softmax函数,通过线性变换和指数函数的组合,将输入向量映射到一个概率分布上。在训练过程中,通过最小化交叉熵损失函数来更新模型参数,从而使得模型能够更好地辨别不同的数字。
相关推荐
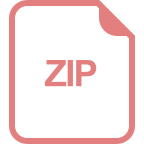












