arcgis用python去除tif文件在shp文件范围外的部分
时间: 2024-09-12 20:04:12 浏览: 64
ArcGIS Python中可以使用 arcpy 和 rasterio 库来处理地理空间数据,包括去除TIFF文件中超出Shapefile范围的部分。这里是一个简化的步骤:
1. 首先,需要安装必要的库,如`arcpy`(ArcGIS的Python API)和`rasterio`,如果尚未安装,可以分别通过命令行运行:
```bash
pip install arcgis rasterio
```
2. 导入所需的模块:
```python
import arcpy
from rasterio import open
```
3. 打开Shapefile和TIFF文件:
```python
# Shapefile路径
shapefile = "your_shapefile.shp"
# TIFF文件路径
tiff_file = "your_tiff.tif"
# 使用arcpy读取shapefile的几何信息
with arcpy.da.SearchCursor(shapefile, ["SHAPE@"]) as cursor:
shapes = [row[0] for row in cursor]
# 使用rasterio读取TIF数据
with open(tiff_file, 'r') as src:
tif_data = src.read()
transform = src.transform
```
4. 根据Shapefile的几何信息裁剪TIFF:
```python
clipped_data = []
for shape in shapes:
bounds = shape.extent
window = ((bounds.XMin, bounds.YMax), (bounds.XMax, bounds.YMin)) # 由于rasterio窗口是左下右上,所以需要交换Y轴
cropped_image = tif_data[window]
clipped_data.append(cropped_image)
```
5. 最后,将裁剪后的图像保存为新的TIFF文件:
```python
output_dir = "output_clipped/"
if not os.path.exists(output_dir):
os.makedirs(output_dir)
for i, cropped in enumerate(clipped_data):
out_tif_name = f"{output_dir}clipped_{i}.tif"
with rasterio.open(out_tif_name, 'w', driver='GTiff', height=cropped.shape[1], width=cropped.shape[2],
transform=transform, crs=tif_data.crs, count=1, dtype=rasterio.float64) as dst:
dst.write_band(1, cropped)
```
阅读全文
相关推荐
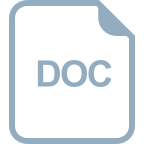
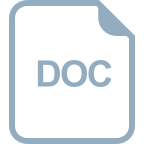
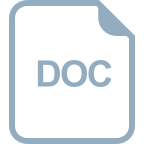
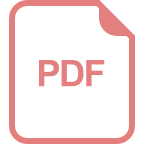
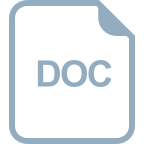


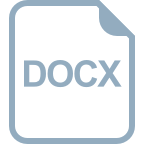
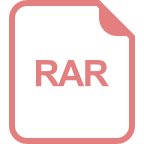
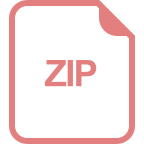
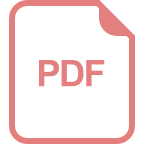
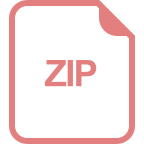
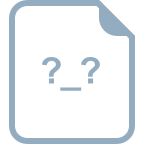
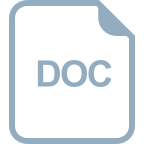




